In this tutorial I would like to show you how easily you can evaluate and display SLAMTEC's RPLiDAR as a plot using Python. As a result, a window should be displayed that shows the current LiDAR values.
The test environment in this example is a cardboard box. But you can also create a different environment. But note that for a larger environment you have to adjust the Python constant D_MAX: int = 500. Outdoors, a value of 4000 is recommended instead of 500.
Note: Prevent the laser from hitting your eyes!

I move the object (unicorn) in the box to see how the capture reacts and is displayed. You can also use multiple objects for your tests!
You need 3 important Python packages/libraries. “matplotlib”, “numpy” and “rplidar-robotica”. It is recommended to install these extensions in a virtual Python environment so that you keep your basic system clean and you can later test with different Python versions.
# create a project directory
$ mkdir ~/RPLiDAR
# change into project directory
$ cd ~/RPLiDAR
# create virtualenvironment
$ python3 -m venv .venv
# activate virtualenvironment
$ source .venv/bin/activate
# install all python dependencies
(.venv) $ pip3 install matplotlib numpy rplidar-roboticia
# list installed dependencies (optional)
(.venv) $ pip3 freeze
Always pay attention to what environment you are in! As soon as you are in the virtual environment, the prompt should show it (example: .venv).
Connect the LiDAR to your computer via USB and search/copy the path.
Example for macOS:
$ ls -la /dev/cu*
/dev/cu.usbserial-0001
Example for Linux:
$ ls -la /dev/ttyUSB*
/dev/ttyUSB0
Note: Depending on the OS and the USB devices already connected, the path may be slightly different for you.
Before we start with the Python script for the plot, we recommend creating another script (named device_information.py) to test whether the LiDAR device really works and whether a connection via Python is possible. Additionally, important information about the device is displayed. Depending on the system and path, adjust the constants MAC_DEVICE_PATH, LINUX_DEVICE_PATH and DEVICE_PATH before running the script.
from os import path
from datetime import datetime
from rplidar import RPLidar
BAUD_RATE: int = 115200
TIMEOUT: int = 1
MAC_DEVICE_PATH: str = '/dev/cu.usbserial-0001'
LINUX_DEVICE_PATH: str = '/dev/ttyUSB0'
DEVICE_PATH: str = MAC_DEVICE_PATH
if __name__ == '__main__':
if path.exists(DEVICE_PATH):
print(f'Found RPLidar on path: {DEVICE_PATH}')
now = datetime.now()
dt_string = now.strftime("%d/%m/%Y %H:%M:%S")
print(f'Date and time: {dt_string}')
lidar = RPLidar(port=DEVICE_PATH, baudrate=BAUD_RATE, timeout=TIMEOUT)
info = lidar.get_info()
for key, value in info.items():
print(f'{key.capitalize()}: {value}')
health = lidar.get_health()
print(f'Health: {health}')
lidar.stop()
lidar.stop_motor()
lidar.disconnect()
else:
print(f'No device found for: {DEVICE_PATH}')
If everything worked and you are satisfied with the result, create the final script. In this example it is named "plot.py".
from os import path
from sys import exit
import matplotlib.animation as animation
import matplotlib.pyplot as plt
import numpy as np
from rplidar import RPLidar
BAUD_RATE: int = 115200
TIMEOUT: int = 1
MAC_DEVICE_PATH: str = '/dev/cu.usbserial-0001'
LINUX_DEVICE_PATH: str = '/dev/ttyUSB0'
DEVICE_PATH: str = MAC_DEVICE_PATH
D_MAX: int = 500
I_MIN: int = 0
I_MAX: int = 50
def verify_device() -> bool:
if path.exists(DEVICE_PATH):
return True
else:
return False
def update_line(num, iterator, line):
scan = next(iterator)
offsets = np.array([(np.radians(meas[1]), meas[2]) for meas in scan])
line.set_offsets(offsets)
intents = np.array([meas[0] for meas in scan])
line.set_array(intents)
return line
if __name__ == '__main__':
if not verify_device():
print(f'No device found: {DEVICE_PATH}')
exit(1)
lidar = RPLidar(port=DEVICE_PATH, baudrate=BAUD_RATE, timeout=TIMEOUT)
lidar.start_motor()
lidar.start_motor()
try:
plt.rcParams['toolbar'] = 'None'
fig = plt.figure()
ax = plt.subplot(111, projection='polar')
line = ax.scatter([0, 0], [0, 0], s=5, c=[I_MIN, I_MAX], cmap=plt.cm.Greys_r, lw=0)
ax.set_rmax(D_MAX)
ax.grid(True)
iterator = lidar.iter_scans(max_buf_meas=5000)
ani = animation.FuncAnimation(fig, update_line, fargs=(iterator, line), interval=50, cache_frame_data=False)
plt.show()
except KeyboardInterrupt:
lidar.stop()
lidar.stop_motor()
lidar.disconnect()
After the command:
(.venv) $ python3 plot.py
After a few seconds you should see another window in which a plot with the recognized values is continuously displayed.

End the running program using the [ctrl] + [c] keys. Terminate the virtual environment with the following command:
(.venv) $ deactivate
Expand the program as you wish and develop great projects yourself using the basic knowledge.
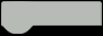