DIY an Arduino Weather Station with Real-Time Display Using 2.0 Inch LVGL ESP32-S3 IPS Screen
Introduction
In this project, we'll create a sleek and functional Arduino weather station using the Arduino UNO and various meteorological sensors. Equipped with a 2.0 Inch LVGL ESP32-S3 IPS Screen, this weather station offers real-time data on temperature, humidity, pressure, and UV levels. Its clean icons, dynamic effects, and text display ensure an elegant visual experience. This mini weather station is perfect for home automation and STEM education projects.

What you can learn from this project:
- Sensor Integration: Learn how to use GPIO pins to connect and configure sensors and how to read and process the data.
- Data Visualization: Integrate sensor data on a display screen, visually presenting weather information using text, icons, widgets and so on.
- Interface Design: Design a user-friendly weather station interface, optimizing response speed and user experience.
- Programming and Debugging: Write code in the Arduino language to control sensors and the display, and master debugging techniques to ensure smooth project operation.
When choosing the display, I have the following requirements: the screen should be small, high resolution, clear display, support for graphics and dynamic styles, and easy to use, adjust and modify. After some comparison, I found that the DFRobot 2.0" color IPS display fully met these requirements.
1. Clear Display: With a 320×240 high resolution and IPS technology, it ensures sharp images, even when viewed from different angles.
2. Easy Interface Design: It supports the LVGL library, making interface design much simpler. Whether you're creating icons or animations, it's easily achievable.
3. Great Compatibility: It offers both I2C and UART interfaces, making it adaptable to various controllers and easy to connect.
4. Built-In Powerful Features: It supports graphics and animations display, saving a lot of extra work.
5. Quick to Start: The official wiki provides Arduino libraries and sample code, so even beginners can quickly get started.
Software
- Download Arduino IDE: Click here to download Arduino IDE
- Download Arduino library: Click here to download DFRobot_LcdDisplay library.
- Click here to see the tutorial: How to install a library?
Hardware Connection
Connections and interfaces according to the following diagram.
Project Process
Here are the key parts of the project. You can check it with the code together at the end of the article.
1. Import Library
Import essential libraries for managing the display, sensors, and communication functionalities.
2. Variable Definitions & Timer Interruptions
Variable Definitions: Store sensor data (e.g., temperature, humidity, barometric pressure, UV intensity) and manage display-related variables like time and label ID.
Timer Interruptions: Manage time increments, resetting seconds and minutes as needed.
3. Initialization and Display Configuration
- LCD Display Initialization: Set up the LCD screen, including background color, screen clearing, and timing.
- Timer Setup: Schedule regular updates to the displayed time and start the timer.
- Display Time and Date: Show the current time and date on specific screen coordinates.
- Icon Display: Position an icon (e.g., “Cloudy.gif”) on the screen.
4. Add Icons
Implement icons from a USB flash drive, allowing customization of position and size. In this project, we'll create four sensors—UV, temperature, humidity, and barometric pressure—along with six plant icons. The following code demonstrates how to achieve this:

5. Add Bar Function
Create and customize progress bars to visualize data from sensors. In this project, we'll create four progress bars, one for each sensor, to show the changes in their data.

6. Read and display data
Read data from each sensor, including temperature, humidity, barometric pressure and UV intensity. Updates the read data in real time on the LCD screen in the form of progress bars and labels.
Code
#include "DFRobot_LcdDisplay.h"
#include <MsTimer2.h>
DFRobot_Lcd_IIC lcd(&Wire, /*I2CAddr*/ 0x2c);
//LTR390 UV Sensor
#include "DFRobot_LTR390UV.h"
#include <SoftwareSerial.h>
#define UARTMODE //UART mode
SoftwareSerial mySerial(/*rx =*/4, /*tx =*/5);
DFRobot_LTR390UV ltr390(/*addr =*/LTR390UV_DEVICE_ADDR, /*s =*/&mySerial);
//BMP388 Pressure Sensor
#include <DFRobot_BMP3XX.h>
DFRobot_BMP388_I2C sensor(&Wire, sensor.eSDOVDD);
#define CALIBRATE_ABSOLUTE_DIFFERENCE
//DHT11 Temperature and Humidity Sensor
#include <dht11.h>
dht11 DHT;
#define DHT11_PIN 10
uint8_t Tem, Hum, Pre, Ult, lcdTimeId,labelId1,labelId2,labelId3,labelId4;
uint8_t hour = 9, Minute = 8, second = 56;
uint8_t lastSecond = 0;
void flash() //Interrupt processing program for time change
{
second++;
if (second > 59) {
second = 0;
Minute++;
}
if (Minute > 59) {
Minute = 0;
hour++;
}
}
void setup(void){
lcd.begin();
lcd.setBackgroundColor(WHITE);
lcd.cleanScreen();
delay(500);
MsTimer2::set(1000, flash); // Set the interrupt function, enter the interrupt every 1000ms
MsTimer2::start(); // Start counting
lcdTimeId = lcd.drawLcdTime(10, 90, hour, Minute, second, 1, LIGHTGREY); //Display the current time
lcd.drawLcdDate(10, 120, 4, 7, 7, 0, LIGHTGREY); //Display the current date
lcd.drawGif(20, 5, "Cloudy.gif", 512); //Create an icon
//Create icons for corresponding sensors
lcd.drawIcon(124, -8, "/sensor icon/thermometer.png", 120);
lcd.drawIcon(119, 35, "/sensor icon/raindrops.png", 120);
lcd.drawIcon(110, 78, "/sensor icon/pressure.png", 120);
lcd.drawIcon(109, 118, "/season icon/sunny.png", 120);
//Create multiple icons
lcd.drawIcon(0, 176, "/botany icon/Potted plant flower.png", 256);
lcd.drawIcon(53, 176, "/botany icon/cactus3.png", 256);
lcd.drawIcon(106, 176, "/botany icon/grass.png", 256);
lcd.drawIcon(159, 176, "/botany icon/grass1.png", 256);
lcd.drawIcon(212, 176, "/botany icon/cactus1.png", 256);
lcd.drawIcon(265, 176, "/botany icon/cactus2.png", 256);
//Create progress bars to display temperature
Tem = lcd.creatBar(164, 22, 80, 15, ORANGE);
labelId1 = lcd.drawString( 255, 22, "0°C", 0, ORANGE);
//Create progress bars to display humidity
Hum = lcd.creatBar(164, 62, 80, 15, RED);
labelId2 = lcd.drawString( 255, 62, "0%", 0, RED);
//Create progress bars to display pressure
Pre = lcd.creatBar(164, 102, 80, 15, BLUE);
labelId3 = lcd.drawString( 255, 102, "0Pa", 0, BLUE);
//Create progress bars to display UV intensity
Ult = lcd.creatBar(164, 142, 80, 15, GREEN);
labelId4 = lcd.drawString( 255, 142, "0lux", 0, GREEN);
//LTR390 UV Sensor initialization settings
#define UARTMODE
mySerial.begin(9600);
Serial.begin(115200);
while(ltr390.begin() != 0){
Serial.println(" Sensor initialize failed!!");
delay(1000);
}
Serial.println(" Sensor initialize success!!");
ltr390.setALSOrUVSMeasRate(ltr390.e18bit,ltr390.e100ms);//18-bit data, sampling time 100ms
ltr390.setALSOrUVSGain(ltr390.eGain3);//3x gain
ltr390.setMode(ltr390.eALSMode);//Set UV mode
//BMP388 Pressure Sensor initialization settings
int rslt;
while( ERR_OK != (rslt = sensor.begin()) ){
if(ERR_DATA_BUS == rslt){
Serial.println("Data bus error!!!");
}else if(ERR_IC_VERSION == rslt){
Serial.println("Chip versions do not match!!!");
}
delay(3000);
}
Serial.println("Begin ok!");
while( !sensor.setSamplingMode(sensor.eUltraPrecision) ){
Serial.println("Set samping mode fail, retrying....");
delay(3000);
}
delay(100);
#ifdef CALIBRATE_ABSOLUTE_DIFFERENCE
if( sensor.calibratedAbsoluteDifference(540.0) ){
Serial.println("Absolute difference base value set successfully!");
}
#endif
float sampingPeriodus = sensor.getSamplingPeriodUS();
Serial.print("samping period : ");
Serial.print(sampingPeriodus);
Serial.println(" us");
float sampingFrequencyHz = 1000000 / sampingPeriodus;
Serial.print("samping frequency : ");
Serial.print(sampingFrequencyHz);
Serial.println(" Hz");
delay(1000);
}
void loop(void){
if(lastSecond != second){
lastSecond = second;
lcd.updateLcdTime(lcdTimeId, 10, 90, hour, Minute, lastSecond, 1, LIGHTGREY); //Display the current time
}
delay(100);
uint32_t date4 = 0;
date4 = ltr390.readALSTransformData(); //Get the transformed data of ambient light
float date3 = sensor.readPressPa(); //Get the pressure data of the pressure sensor
DHT.read(DHT11_PIN); //Get the temperature and humidity data of the temperature and humidity sensor
Serial.println("-------------");
Serial.println(DHT.temperature);
Serial.println(DHT.humidity);
Serial.println(date3);
Serial.println(date4);
//Set the value of the progress bars
lcd.setBarValue(Tem, DHT.temperature);
lcd.updateString(labelId1, 255, 22, String(DHT.temperature)+"°C", 0, ORANGE);
delay(100);
lcd.setBarValue(Hum, DHT.humidity);
lcd.updateString(labelId2, 255, 62, String(DHT.humidity)+"%", 0, RED);
delay(100);
lcd.setBarValue(Pre, date3);
lcd.updateString(labelId3, 255, 102, String(date3)+"Pa", 0, BLUE);
delay(100);
lcd.setBarValue(Ult, date4);
lcd.updateString(labelId4, 255, 142, String(date4)+"lux", 0, GREEN);
delay(100);
}
Done!
In this project, we have successfully built a fully functional weather station that not only displays various weather data but also delivers a visually appealing and intuitive interface. Through this project, you’ve learned how to integrate sensors and process data, as well as how to visualize data and design interfaces using the LVGL library.
Looking ahead, this project can be further expanded and optimized by adding more types of sensors or by uploading weather data to the cloud for remote monitoring. We hope that this project has sparked your creativity and inspired you to continue exploring and innovating, leading to the development of more interesting and practical electronic projects.

You can also customize it to your liking with a black background, as shown below.
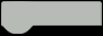