Hey there, future Bluetooth Jedi! 🌟 Welcome to an electrifying project where we combine the magic of Web Bluetooth, the flashy brilliance of NeoPixels, and the awesome power of the Seeed Studio Xiao nRF52840 Sense. By the end of this journey, you'll be able to control a strip of NeoPixels right from your web browser. Yep, no more complicated apps or cables—just pure wireless awesomeness. 🎮✨
So, grab your soldering iron, a cup of coffee (or tea ☕), and let’s light things up! 💥
🛠️ What You’ll Need:
Before we dive into code and soldering, let's check if you have all the necessary gadgets to make this magic happen!
1. Seeed Studio Xiao nRF52840 Sense ⚡
Why this? It’s like a pocket-sized superhero! 💪 It's BLE-capable, has a built-in IMU, microphone, and is powered by the ARM Cortex-M4 processor. Small but mighty!
2. NeoPixel LED Strip 🌈
These RGB LEDs are the stars of our show. They can display millions of colors and are individually addressable. We’ll be using them to dazzle our friends (or just our cat 🐱).
3. Web Browser with Bluetooth Support 🌐
For this project, we need a web browser that supports Web Bluetooth API. Chrome, Edge, or Chromium-based browsers are perfect. Sorry, Firefox lovers... you'll have to switch sides for this one! 😅
4. Jumper Wires, Soldering Kit, and USB-C Cable 🔌
Standard build-essentials. These will help you hook up everything without blowing things up (which we totally don’t want).
Get PCBs for Your Projects Manufactured
You must check out PCBWAY for ordering PCBs online for cheap!
You get 10 good-quality PCBs manufactured and shipped to your doorstep for cheap. You will also get a discount on shipping on your first order. Upload your Gerber files onto PCBWAY to get them manufactured with good quality and quick turnaround time.
PCBWay now could provide a complete product solution, from design to enclosure production. Check out their online Gerber viewer function. With reward points, you can get free stuff from their gift shop. Also, check out this useful blog on PCBWay Plugin for KiCad from here. Using this plugin, you can directly order PCBs in just one click after completing your design in KiCad.
Step 1: Setting Up Your Xiao nRF52840 Sense 📦
First, we need to make sure your Xiao is ready for action. Time to upload some code!
Install the Development Environment:
Install Arduino IDE from the official website if you already have it. Next, install the Seeed nRF52 boards in Arduino: Go to File > Preferences. Add this URL to Additional Boards Manager URLs: https://files.seeedstudio.com/arduino/package_seeeduino_boards_index.jsonNow head to Tools > Board > Boards Manager, search for Seeed nRF52 and install the package.
Install Libraries:
You’ll need to grab a couple of libraries to work with NeoPixels and BLE:
Adafruit NeoPixel Library (to handle our shiny lights 💡) ArduinoBLE Library (for Bluetooth communication)
Head to Tools > Manage Libraries and search for these to install them.
Step 2: Wiring it Up 🧑🔧🔌
Alright, it's time to connect the Xiao to the NeoPixel strip. Don’t worry, this part is easier than figuring out which wire your headphones use! 🎧
Connect the NeoPixels to Xiao:
Power (VCC): Connect this to the 3.3V pin on the Xiao.Ground (GND): GND to GND (these two are like peanut butter and jelly 🥪—inseparable).Data In (DIN): Hook this up to Pin D0 on the Xiao.
Everything wired up? Awesome! Now the fun begins. 🧙♂️✨
Step 3: Code Time! ⌨️💻
Let's dive into the code that will make your Xiao and NeoPixels dance to your commands (remotely via Bluetooth!). 🕺💃
Here's the plan:
The Xiao will advertise itself as a Bluetooth device.Your browser (with Web Bluetooth) will connect to it and control the NeoPixel strip by sending color commands.
Here's the Arduino Sketch:
#include <ArduinoBLE.h>
#include <Adafruit_NeoPixel.h>
#define NEOPIXEL_PIN 0
#define NUM_PIXELS 64
Adafruit_NeoPixel strip = Adafruit_NeoPixel(NUM_PIXELS, NEOPIXEL_PIN, NEO_GRB + NEO_KHZ800);
BLEService ledService("19b10000-e8f2-537e-4f6c-d104768a1214");
BLEByteCharacteristic ledCharacteristic("19b10001-e8f2-537e-4f6c-d104768a1214", BLEWrite | BLERead);
void setup() {
Serial.begin(115200);
if (!BLE.begin()) {
Serial.println("starting BLE failed!");
while (1);
}
BLE.setLocalName("XIAO-LED");
BLE.setAdvertisedService(ledService);
ledService.addCharacteristic(ledCharacteristic);
BLE.addService(ledService);
ledCharacteristic.writeValue(0);
strip.begin();
strip.show();
BLE.advertise();
Serial.println("Bluetooth device active, waiting for connections...");
}
void loop() {
BLEDevice central = BLE.central();
if (central) {
Serial.print("Connected to central: ");
Serial.println(central.address());
while (central.connected()) {
if (ledCharacteristic.written()) {
uint8_t ledState = ledCharacteristic.value();
if (ledState == 1) {
digitalWrite(LED_BUILTIN, HIGH); // Turn LED on
for (int i = 0; i < NUM_PIXELS; i++) {
strip.setPixelColor(i, strip.Color(255, 255, 255));
strip.show();
}
} else {
digitalWrite(LED_BUILTIN, LOW); // Turn LED off
for (int i = 0; i < NUM_PIXELS; i++) {
strip.setPixelColor(i, strip.Color(0, 0, 0));
strip.show();
}
}
}
}
Serial.print("Disconnected from central: ");
Serial.println(central. Address());
}
}
What Does This Code Do? 🧐
BLE Advertising: Your Xiao advertises itself as "NeoPixelController" via Bluetooth. BLE Service: We create a custom Bluetooth service that listens for commands (color changes) from a web browser. NeoPixel Control: Based on the color data sent by the browser, the NeoPixel LEDs change colors accordingly.
Step 4: Build the Web Bluetooth Interface 🌐
Now we move to the browser side, creating a simple webpage that will scan for your Xiao and send color commands via Bluetooth.
Here’s a basic HTML + JavaScript setup
<!DOCTYPE html>
<html>
<head>
<title>Web Bluetooth LED Control</title>
</head>
<body>
<button id="connect">Connect</button>
<button id="on">Turn On</button>
<button id="off">Turn Off</button>
<script>
let ledCharacteristic;
document.getElementById('connect').addEventListener('click', async () => {
try {
const device = await navigator.bluetooth.requestDevice({
filters: [{ name: 'XIAO-LED' }],
optionalServices: ['19b10000-e8f2-537e-4f6c-d104768a1214']
});
const server = await device.gatt.connect();
const service = await server.getPrimaryService('19b10000-e8f2-537e-4f6c-d104768a1214');
ledCharacteristic = await service.getCharacteristic('19b10001-e8f2-537e-4f6c-d104768a1214');
console.log('Connected to device');
} catch (error) {
console.error('Error:', error);
}
});
document.getElementById('on').addEventListener('click', async () => {
if (ledCharacteristic) {
try {
await ledCharacteristic.writeValue(Uint8Array.of(1));
console.log('LED turned on');
} catch (error) {
console.error('Error turning on LED:', error);
}
}
});
document.getElementById('off').addEventListener('click', async () => {
if (ledCharacteristic) {
try {
await ledCharacteristic.writeValue(Uint8Array.of(0));
console.log('LED turned off');
} catch (error) {
console.error('Error turning off LED:', error);
}
}
});
</script>
</body>
</html>
How This Works:
The page contains a button to connect to the Xiao via Bluetooth. Once connected, you can use the on and off buttons to control the LED.
Step 5: Time to Shine! ✨💡
Now for the moment of truth: test your project! 🚀
Upload the Arduino code to your Xiao nRF52840 Sense.Open the HTML file in a supported browser (like Chrome).Click the "Connect to Xiao" button, and select the device from the Bluetooth device list.Slide the color picker, and watch the magic happen! 🪄✨ Your NeoPixels should change colors as you adjust the slider. Wireless LED control, baby!
Step 6: Add Your Own Magic ✨
Congrats, you've just controlled a strip of NeoPixels via Web Bluetooth! 🎉 But why stop there? Here are some ways to level up:
Add color animations: How about some rainbow patterns or breathing effects? 🌈IMU Integration: Use the onboard IMU to control the lights based on motion. You can dance and let the LEDs react to your moves! 🕺Build a Web Dashboard: Make the webpage more interactive by adding buttons for pre-defined light patterns (disco mode, anyone?).
Conclusion: The Wireless Wonderland 🎆
With Web Bluetooth and the Xiao nRF52840, you’ve unlocked the secret to controlling LEDs without touching a wire! 🎮 Whether you're jazzing up your living room, building smart home gadgets, or just flexing at your next tech meetup, you're officially in control of the light show! 👨💻👩💻
So go on, experiment, and make your project shine brighter than a disco ball at a retro party! 💃✨
Happy hacking, and keep shining! 🌟
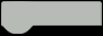