USB Wio - E5 with Python Will guide you to integrate Wio E5 with python script.
Story
This comprehensive tutorial will guide you through integrating the powerful LoRa Wio E5 module with a Windows PC using Python and a USB to TTL converter. This setup will enable seamless communication with the Wio E5 module via serial communication, allowing you to send AT commands and receive responses effortlessly. 💻🔌📡
By the end of this tutorial, you’ll have a fully functional setup and gain insights into various creative applications and projects you can build using this integration. Whether you’re looking to develop IoT solutions, environmental monitoring systems, or remote control applications, this guide will provide you with the foundational knowledge and inspiration to get started. 🌍📈🔧
Components Needed
LoRa Wio E5 module 📡 USB to TTL converter 🔌 Jumper wires 🧵 Windows PC 💻 Python installed on your PC 🐍 USB cable 🔋Step 1: Hardware Connections
Connect the Wio E5 to the USB to TTL Converter:
Wio E5 VCC to USB to TTL VCC Wio E5 GND to USB to TTL GND Wio E5 TX to USB to TTL RX Wio E5 RX to USB to TTL TXConnect the USB to the TTL Converter to your PC:
Plug the USB to TTL converter into a USB port on your Windows PC.
Step 2: Install Python and PySerial
Download and Install Python:
Download Python from the official Python website. Follow the installation instructions to install Python on your Windows PC.Install PySerial:
Open Command Prompt and run the following command to install the PySerial library:
pip install pyserial
Step 3: Identify the COM Port
Find the COM Port:
Open Device Manager on your Windows PC. Expand the “Ports (COM & LPT)” section. Note the COM port number assigned to your USB to TTL converter (e.g., COM3).
Step 4: Write the Python Code
Create a Python Script:
Open your preferred text editor or IDE and create a new Python script (e.g., lora_wio_e5.py).Write the Code:
Copy and paste the following codes into your Python script:
## Transmitter Script:-
import serial
import time
# Definition of the timeout
read_timeout = 0.2
# Create the instance to manage the board via the serial port
lora = serial.Serial(
port='COM23',
baudrate=9600,
bytesize=8,
parity='N',
timeout=1,
stopbits=1,
xonxoff=False,
rtscts=False,
dsrdtr=False
)
def envoi_test_reponse(chaine_verif, timeout_ms, commande):
startMillis = int(round(time.time() * 1000))
# Check if the verification string exists
if chaine_verif == "":
return 0
# Send the command
fin_ligne = "\r\n"
cmd = "%s%s" % (commande, fin_ligne)
print("Command sent = ", cmd)
lora.write(cmd.encode())
# Wait for the response
reponse = ""
quantity = lora.in_waiting
# Read the response from the board until timeout
while int(round(time.time() * 1000)) - startMillis < timeout_ms:
# If we have data
if quantity > 0:
# Add them to the response string
reponse += lora.read(quantity).decode('utf-8')
print("Response from the board: ", reponse)
else:
# Otherwise wait a moment
time.sleep(read_timeout)
# Check if we have received data
quantity = lora.in_waiting
print("Response from the board: ", reponse)
# Check if the expected string exists
if chaine_verif in reponse:
print("The response string exists", reponse)
return 1
else:
return 0
if envoi_test_reponse("+AT: OK", 1000, "AT"):
envoi_test_reponse("+MODE: TEST", 1000, "AT+MODE=TEST")
while True:
temp1 = 25 # Replace with your first temperature value
temp2 = 30 # Replace with your second temperature value
payload1 = '{:04x}'.format(temp1)
payload2 = '{:04x}'.format(temp2)
combined_payload = payload1 + payload2
# Use the combined payload in the function call
envoi_test_reponse("AT+TEST=TXLRPKT", 1000, f"AT+TEST=TXLRPKT, {combined_payload}")
# envoi_test_reponse("AT+TEST=TXLRPKT", 1000, "AT+TEST=TXLRPKT, AB")
time. Sleep(1)
## Reciever Script :-
# Import the libraries
import serial
import time
# Definition of flags
is_join = False # Can join the board
is_exist = False # The Wio E5 board has been detected
# Definition of the timeout
read_timeout = 0.2
# Create the instance to manage the board via the serial port
lora = serial.Serial(
port='COM3',
baudrate=9600,
bytesize=8,
parity='N',
timeout=1,
stopbits=1,
xonxoff=False,
rtscts=False,
dsrdtr=False
)
def envoi_test_reponse(chaine_verif, timeout_ms, commande):
startMillis = int(round(time.time() * 1000))
# Check if the verification string exists
if chaine_verif == "":
return 0
# Send the command
fin_ligne = "\r\n"
cmd = "%s%s" % (commande, fin_ligne)
print("Command sent = ", cmd)
lora.write(cmd.encode())
# Wait for the response
reponse = ""
quantity = lora.in_waiting
# Read the response from the board until timeout
while int(round(time.time() * 1000)) - startMillis < timeout_ms:
# If we have data
if quantity > 0:
# Add them to the response string
reponse += lora.read(quantity).decode('utf-8')
print("Response from the board: ", reponse)
else:
# Otherwise wait a moment
time.sleep(read_timeout)
# Check if we have received data
quantity = lora.in_waiting
print("Response from the board: ", reponse)
# Check if the expected string exists
if chaine_verif in reponse:
print("The response string exists", reponse)
return 1
else:
return 0
# Board configuration
if envoi_test_reponse("+AT: OK", 1000, "AT"):
# The board has been detected = set to True
envoi_test_reponse("+MODE: TEST", 1000, "AT+MODE=TEST")
while True:
envoi_test_reponse("+TEST: RX '0019001E'", 1000, "AT+TEST=RXLRPKT")
time. Sleep(1)
Save the Script:
Save the script with a .py extension (e.g., lora_wio_tx_e5.py, lora_wio_rx_e5.py).
Step 5: Run the Python Script
Execute the Script:
Open Command Prompt. Navigate to the directory where your script is saved.Run the script using the following command:
python lora_wio_tx_e5.py
python lora_wio_rx_e5.py
The script will send AT commands to the Wio E5 module and print the responses in the Command Prompt.
Troubleshooting Tips
Check Connections: Ensure all connections are secure and correct. Verify COM Port: Double-check the COM port number in the Device Manager and update the script if necessary. Permissions: Ensure you have the necessary permissions to access the COM port. Error Messages: Pay attention to any error messages in the Command Prompt for clues on what might be wrong.
Conclusion
🎉 Congratulations! 🎉 You have successfully integrated the LoRa Wio E5 module with your Windows PC using Python and a USB to TTL converter. This setup allows you to send AT commands to the Wio E5 module and receive responses, opening up a world of possibilities for developing various IoT applications using LoRa communication. 🌐
Happy coding and innovating! 💻📡✨
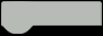