Introduction
In this project, we will build a low-power environmental logger using the DFRobot Beetle ESP32-C6, a multifunctional environmental sensor from DFRobot, and the Qubitro IoT platform. The ESP32-C6's deep sleep feature will minimize power consumption, making it ideal for battery-powered applications.
Ā
Ā
Ā
Components Needed
DFRobot Beetle ESP32-C6: A compact development board with Wi-Fi 6, Bluetooth 5, Zigbee 3.0, and Thread 1.3 support.
DFRobot Multifunctional Environmental Sensor: Combines temperature, humidity, atmospheric pressure, ambient light, and UV sensors into one module.Qubitro IoT Platform: An end-to-end cloud platform for building and operating scalable connected experiences.Breadboard and jumper wiresPower supply or battery
Ā
Get PCBs for Your Projects Manufactured
Ā
Ā
You must check out PCBWAY for ordering PCBs online for cheap!
Ā
You get 10 good-quality PCBs manufactured and shipped to your doorstep for cheap. You will also get a discount on shipping on your first order. Upload your Gerber files onto PCBWAY to get them manufactured with good quality and quick turnaround time. PCBWay now could provide a complete product solution, from design to enclosure production. Check out their online Gerber viewer function. With reward points, you can get free stuff from their gift shop. Also, check out this useful blog on PCBWay Plugin for KiCad from here. Using this plugin, you can directly order PCBs in just one click after completing your design in KiCad.
Ā
About the DFRobot Beetle ESP32-C6
The DFRobot Beetle ESP32-C6 is a powerful and compact development board designed for IoT applications. It features the latest Wi-Fi 6 technology, which offers improved performance and efficiency compared to previous Wi-Fi standards. Additionally, it supports Bluetooth 5, Zigbee 3.0, and Thread 1.3, making it a versatile choice for various wireless communication needs.
Ā
Key features of the Beetle ESP32-C6 include:
Wi-Fi 6: Enhanced speed, capacity, and power efficiency.Bluetooth 5: Improved range and data transfer rates.Zigbee 3.0 and Thread 1.3: Reliable and secure mesh networking protocols.Compact Size: Ideal for space-constrained projects.Low Power Consumption: Perfect for battery-powered applications.Ā
Ā
Ā
About the DFRobot Multifunctional Environmental Sensor
The DFRobot Multifunctional Environmental Sensor is an all-in-one module that combines multiple sensors to measure various environmental parameters. This sensor is ideal for projects that require comprehensive environmental monitoring.
Key features of the DFRobot Multifunctional Environmental Sensor include:
Temperature Sensor: Measures ambient temperature.Humidity Sensor: Measures relative humidity.Atmospheric Pressure Sensor: Measures barometric pressure.Ambient Light Sensor: Measures light intensity.UV Sensor: Measures ultraviolet light levels.Ā
Ā
Ā
Step 1: Setting Up the Hardware
Connect the multifunctional environmental sensor to the Beetle ESP32-C6 using the I2C communication protocol. This protocol allows multiple sensors to connect to just two pins on the microcontroller â the SDA (Serial Data Line) and SCL (Serial Clock Line).
Ā
Ā
Ensure that the connections are secure and that each sensorâs SDA and SCL pins are correctly connected to the corresponding pins on the Beetle ESP32-C6.
Ā
Ā
Ā
About the Qubitro IoT Platform
Ā
Qubitro is an end-to-end cloud platform designed for building and operating scalable connected experiences. It provides a comprehensive suite of tools for managing IoT devices, collecting and processing data, and visualizing information through customizable dashboards.
Ā
Ā
Key features of the Qubitro IoT Platform include:
Ā
No-Code Integrations: Easily connect and sync devices and their data from multiple sources without writing code.Real-Time Data Processing: Collect, process, and activate device data in real-time.Customizable Dashboards: Create and customize dashboards to monitor and analyze device data.Scalable Infrastructure: Manage IoT deployments efficiently, from a single device to thousands.
Ā
Integrating the ESP32-C6 with Qubitro
Ā
To integrate the ESP32-C6 with the Qubitro platform, follow these steps:
Create a Qubitro Account: Sign up for a Qubitro account on the Qubitro website.Create a New Project: In the Qubitro portal, create a new project to manage your devices and data.Obtain Qubitro Token: Generate a token for your project, which will be used to authenticate your device.Ā
Ā Install Qubitro Library: Install the Qubitro library in the Arduino IDE to enable communication between the ESP32-C6 and Qubitro.Configure Device: Use the Qubitro library to configure your ESP32-C6 to send data to the Qubitro platform.Ā
Ā
Step 2: Programming the Beetle ESP32-C6
Ā
Using the Arduino IDE, write a program that initializes each sensor and reads data regularly. Libraries for the sensor are available online and can simplify this process. Here is a sample code snippet to get you started:
#include <QubitroMqttClient.h>
#include <WiFi.h>
#include "DFRobot_EnvironmentalSensor.h"
int led = 15;
#include <esp_wifi.h>
#include "driver/adc.h"
DFRobot_EnvironmentalSensor environment(/*addr = */SEN050X_DEFAULT_DEVICE_ADDRESS, /*pWire = */&Wire);
// WiFi Client
WiFiClient wifiClient;
// Qubitro Client
QubitroMqttClient mqttClient(wifiClient);
// Device Parameters
char deviceID[] = "";
char deviceToken[] = "";
// WiFi Parameters
const char* STA_SSID = "";
const char* STA_PASS = "";
void setup()
{
Serial.begin(9600);
enableWiFi();
pinMode(led, OUTPUT);
Serial.begin(115200);
while (environment.begin() != 0) {
Serial.println(" Sensor initialize failed!!");
delay(1000);
}
Serial.println(" Sensor initialize success!!");
digitalWrite(led, HIGH);
delay(80);
digitalWrite(led, LOW);
delay(80);
}
void loop()
{
char host[] = "broker.qubitro.com";
int port = 1883;
mqttClient.setId(deviceID);
mqttClient.setDeviceIdToken(deviceID, deviceToken);
Serial.println("Connecting to Qubitro...");
if (!mqttClient.connect(host, port))
{
Serial.print("Connection failed. Error code: ");
Serial.println(mqttClient.connectError());
Serial.println("Visit docs.qubitro.com or create a new issue on github.com/qubitro");
digitalWrite(led, HIGH);
delay(50);
digitalWrite(led, LOW);
delay(50);
}
Serial.println("Connected to Qubitro.");
mqttClient.subscribe(deviceID);
Serial.println("-------------------------------");
Serial.print("Temp: ");
Serial.print(environment.getTemperature(TEMP_C));
Serial.println(" ā");
Serial.print("Temp: ");
Serial.print(environment.getTemperature(TEMP_F));
Serial.println(" ā");
Serial.print("Humidity: ");
Serial.print(environment.getHumidity());
Serial.println(" %");
Serial.print("Ultraviolet intensity: ");
Serial.print(environment.getUltravioletIntensity());
Serial.println(" mw/cm2");
Serial.print("LuminousIntensity: ");
Serial.print(environment.getLuminousIntensity());
Serial.println(" lx");
Serial.print("Atmospheric pressure: ");
Serial.print(environment.getAtmospherePressure(HPA));
Serial.println(" hpa");
Serial.print("Elevation: ");
Serial.print(environment.getElevation());
Serial.println(" m");
Serial.println("-------------------------------");
String payload = "{\"Temp\": " + String(environment.getTemperature(TEMP_C)) + ",\"Humidity\":" + String(environment.getHumidity()) + ",\"LuminousIntensity\":" + String(environment.getLuminousIntensity()) + ",\"Pressure\":" + String(environment.getAtmospherePressure(HPA)) + ",\"UV\":" + String(environment.getUltravioletIntensity()) + ",\"Elevation\":" + String(environment.getElevation()) + "}";
mqttClient.poll();
mqttClient.beginMessage(deviceID);
mqttClient.print(payload);
mqttClient.endMessage();
digitalWrite(led, HIGH);
delay(80);
digitalWrite(led, LOW);
delay(80);
digitalWrite(led, HIGH);
delay(80);
digitalWrite(led, LOW);
delay(80);
disableWiFi();
delay(60000);
enableWiFi();
}
void disableWiFi() {
WiFi.disconnect(true); // Disconnect from the network
WiFi.mode(WIFI_OFF); // Switch WiFi off
}
void enableWiFi() {
WiFi.disconnect(false); // Reconnect the network
WiFi.mode(WIFI_STA); // Switch WiFi off
Serial.println("START WIFI");
WiFi.begin(STA_SSID, STA_PASS);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.println("WiFi connected");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
}
Deep Sleep Mode of the ESP32
Ā
The deep sleep mode of the ESP32 is a power-saving feature that allows the microcontroller to enter a low-power state while retaining its ability to wake up and resume operation. This is particularly useful for battery-powered applications where power consumption needs to be minimized.
Key features of the deep sleep mode include:
Low Power Consumption: The ESP32 consumes minimal power while in deep sleep mode.Wake-Up Sources: The ESP32 can be configured to wake up from deep sleep using various sources, such as timers, external interrupts, and touch sensors.Retained Memory: The ESP32 retains its memory contents during deep sleep, allowing it to resume operation quickly upon waking up.
In this project, we use the timer wake-up source to wake up the ESP32 periodically, read sensor data, send it to the Qubitro IoT platform, and then return to deep sleep.
Ā
Ā
Wi-Fi Disable Feature to Reduce Power Consumption
Ā
To further reduce power consumption, we can disable the Wi-Fi module when it is not needed. This can be done by calling the WiFi.disconnect(true) and WiFi.mode(WIFI_OFF) functions. Disabling Wi-Fi helps save power, especially in applications where the device spends most of its time in deep sleep mode.
Ā
Ā
Here is the device's power consumption when connected to the network and sending data to the cloud.
Ā
Ā
The max consumption is about 0.7A for 6 seconds. Then it will be entered into sleep mode by disabling the Wi-Fi.
Ā
Ā
Ā
Creating a Dashboard on Qubitro
Ā
To create a dashboard on Qubitro and visualize your sensor data, follow these steps:
Navigate to Dashboards: In the Qubitro portal, go to the Dashboards tab.Create a New Dashboard: Click on "New Dashboard" and give it a name.Add Widgets: Select the widgets and add the data source.Ā
Ā
Here is the final dashboard.
Ā
Ā
Conclusion:
Ā
Ā
you'll have successfully built a low-power environmental logger using the DFRobot Beetle ESP32-C6, a multifunctional environmental sensor from DFRobot, and the Qubitro IoT platform. Leveraging the ESP32-C6's deep sleep feature ensures minimal power consumption, making it perfect for battery-powered applications. This setup not only provides efficient environmental monitoring but also demonstrates the potential of integrating advanced IoT technologies for sustainable and scalable solutions. Happy logging!
Ā
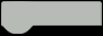