In this extended tutorial, you'll learn how to interface the Seeed LoRa Wio E5 Module with the Arduino UNO and connect your setup to The Things Network (TTN). TTN is a global network that allows IoT devices to connect via LoRaWAN, providing seamless communication for smart city applications, agriculture, and more.
Setting Up The Things Network (TTN)
To interface with TTN, you must create an application, register your device, and get the Device EUI, Application EUI, and App Key.
Step-by-Step Guide to Setting Up TTN:
1. Create a TTN account:
- Sign up at [The Things Network](https://www.thethingsnetwork.org/).
2. Create a new application:
- Go to the TTN Console.
- Navigate to Applications > Add Application.
- Fill in the details like the Application ID and Description.
3. Register your LoRa device:
- Within the created application, go to the End Devices tab and click Add End Device.
- Choose OTAA (Over The Air Activation) as the activation method.
- Fill in the required fields like Device EUI, Application EUI, and App Key.
- If you don’t have a Device EUI, you can generate it on the TTN console or use an existing one (found in your LoRa-E5 documentation).
4. Configure frequency plan:
- Depending on your region, choose the appropriate frequency plan (e.g., EU868, US915, etc.).
5. Save the device information.
Now you have the Device EUI, Application EUI, and App Key from the TTN console, which you’ll need for the next steps.
Get PCBs for Your Projects Manufactured
You must check out PCBWAY for ordering PCBs online for cheap!
You get 10 good-quality PCBs manufactured and shipped to your doorstep for cheap. You will also get a discount on shipping on your first order. Upload your Gerber files onto PCBWAY to get them manufactured with good quality and quick turnaround time. PCBWay now could provide a complete product solution, from design to enclosure production. Check out their online Gerber viewer function. With reward points, you can get free stuff from their gift shop. Also, check out this useful blog on PCBWay Plugin for KiCad from here. Using this plugin, you can directly order PCBs in just one click after completing your design in KiCad.
Hardware Configuration
Connect the LoRa E5 module with Arduino Una via the UART interface. Connect Rx to Arduino 6 and Tx to Arduino 7 and power to 3.3 V.
Configuring Arduino IDE
You already have the Arduino IDE configured for basic LoRa communication. Now, let’s adjust the code to communicate with TTN using the LoRaWAN protocol.
Setting Up LoRaWAN Communication
For LoRaWAN communication with TTN, the Seeed LoRa E5 module uses AT commands to handle the LoRaWAN stack internally. We’ll configure the device to use OTAA (Over Air Activation), where your device joins the network dynamically using the Device EUI, App EUI, and App Key.
Common AT Commands for LoRaWAN:
- AT+MODE=LWOTAA: Set the mode to OTAA.
- AT+ID=DevEui, AppEui: Set the device and application EUI.
- AT+KEY=APPKEY, AppKey: Set the app key.
- AT+JOIN: Join the LoRaWAN network.
- AT+CMSGHEX: Send a message in hexadecimal format.
- AT+PORT=1: Set the port for communication.
Code Example for TTN
The code below will configure your LoRa E5 module to connect to TTN via OTAA.
#include <ArduinoJson.h>
#include<SoftwareSerial.h>
SoftwareSerial e5(7, 6);
static char recv_buf[512];
static int led = 13;
int counter = 0;
//static char recv_buf[512];
static bool is_exist = false;
static bool is_join = false;
static int at_send_check_response(char *p_ack, int timeout_ms, char *p_cmd, ...)
{
int ch;
int num = 0;
int index = 0;
int startMillis = 0;
va_list args;
memset(recv_buf, 0, sizeof(recv_buf));
va_start(args, p_cmd);
e5.print(p_cmd);
Serial.print(p_cmd);
va_end(args);
delay(200);
startMillis = millis();
if (p_ack == NULL)
return 0;
do
{
while (e5.available() > 0)
{
ch = e5.read();
recv_buf[index++] = ch;
Serial.print((char)ch);
delay(2);
}
if (strstr(recv_buf, p_ack) != NULL)
return 1;
} while (millis() - startMillis < timeout_ms);
Serial.println();
return 0;
}
static void recv_prase(char *p_msg)
{
if (p_msg == NULL)
{
return;
}
char*p_start = NULL;
int data = 0;
int rssi = 0;
int snr = 0;
p_start = strstr(p_msg, "RX");
if (p_start && (1 == sscanf(p_start, "RX: \"%d\"\r\n", &data)))
{
Serial.println(data);
if (led)
{
digitalWrite(LED_BUILTIN, LOW);
}
else
{
digitalWrite(LED_BUILTIN, HIGH);
}
}
p_start = strstr(p_msg, "RSSI");
}
void setup(void)
{
Serial.begin(9600);
pinMode(led, OUTPUT);
digitalWrite(led, LOW);
e5.begin(9600);
Serial.print("E5 LOCAL TEST\r\n");
if (at_send_check_response("+AT: OK", 100, "AT\r\n"))
{
is_exist = true;
at_send_check_response("+ID: AppEui", 1000, "AT+ID\r\n");
at_send_check_response("+MODE: LWOTAA", 1000, "AT+MODE=LWOTAA\r\n");
at_send_check_response("+DR: EU868", 1000, "AT+DR=EU868\r\n");
at_send_check_response("+CH: NUM", 1000, "AT+CH=NUM,0-2\r\n");
at_send_check_response("+KEY: APPKEY", 1000, "AT+KEY=APPKEY,\"2B7E151628AED2A6ABF7158809CF4F3D\"\r\n");
at_send_check_response("+CLASS: C", 1000, "AT+CLASS=A\r\n");
at_send_check_response("+PORT: 8", 1000, "AT+PORT=8\r\n");
delay(200);
is_join = true;
}
else
{
is_exist = false;
Serial.print("No E5 module found.\r\n");
}
digitalWrite(led, HIGH);
}
void loop(void)
{
int ret = 0;
if (is_join)
{
ret = at_send_check_response("+JOIN: Network joined", 12000, "AT+JOIN\r\n");
if (ret)
{
is_join = false;
}
else
{
at_send_check_response("+ID: AppEui", 1000, "AT+ID\r\n");
Serial.print("JOIN failed!\r\n\r\n");
delay(5000);
}
}
else
{
char cmd[128];
sprintf(cmd, "AT+CMSGHEX=\"%04X%04X\"\r\n", (int)100, (int)50);
ret = at_send_check_response("Done", 5000, cmd);
digitalWrite(LED_BUILTIN, LOW);
delay(1000);
digitalWrite(LED_BUILTIN, HIGH);
if (ret)
{
recv_prase(recv_buf);
}
else
{
Serial.print("Send failed!\r\n\r\n");
}
delay(2000);
}
}
Explanation:
- Device EUI, App EUI, and App Key are set up according to your TTN registration.
- The module will attempt to join the TTN network using OTAA.
- After joining, it sends the message “100,50” in hexadecimal format.
Testing the Setup with TTN
Once your code is uploaded and the Arduino UNO is powered up, follow these steps to ensure everything is working:
1. Check the Serial Monitor:
- Open the Arduino Serial Monitor to observe the join process.
- You should see a “Join Success” message if everything is set up correctly.
2. Monitor your TTN Console:
- Go to your TTN Console and navigate to the Live Data section of your device.
- You should see the uplink message “100, 50 ” appearing in the data stream.
3. Testing range: Move the device within the range of a nearby TTN gateway to test communication.
Troubleshooting & Tips
- Unable to join TTN: Make sure your Device EUI, App EUI, and App Key are correct. Also, check if you’re within the coverage area of a TTN gateway.
- Antenna setup: Ensure you’ve connected a proper antenna to the LoRa E5 module to get a good signal.
- Check frequency plan: Make sure you’re using the right frequency (EU868, US915, etc.) for your region.
- TTN Console Logs: Monitor logs in the TTN console to see if the device is trying to connect but facing issues.
Conclusion
You’ve successfully interfaced the Seeed LoRa Wio E5 Module with the Arduino UNO and connected it to The Things Network! Now, your device is capable of sending and receiving data via LoRaWAN, making it ideal for building IoT applications such as smart city infrastructure, environmental monitoring, and industrial IoT.
Happy experimenting with TTN!
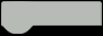