A Big Ben chiming clock using an Arduino Nano, a DS1302 RTC, a DFplayer Mini showing the time on a Nokia 5110 lcd

Things used in this project
Ā
Hardware components
Story
Ā
I became interested in making a chiming clock a few years ago when I had a raspberry pi but although it was successful, it consumed too much power. After getting to know about Arduino's I tried the same project with an RTC and a Dfplayer mini. The current at rest is 20mA.
Ā
The clock is digital and shown on a Nokia 5110 LCD (no backlight as yet). The time is provided by a DS1302 ( i'll assume it is already set to the correct time) and the chime is selected mp3s' from a preloaded micro sdcard on a Dfplayer mini.
Ā
The mp3's are zipped (without a folder) and must be added to an empty micro sdcard in order 0001.mp3 first 0002.mp3 second and so on. This is because the program uses position rather than file names to play the correct chime at the correct time. Although this is only a feature I have used and can easily be altered.
Ā
I have set the (Big Ben) chimes to start playing 20 seconds before each hour between 6am and 10pm (easily altered). This is so the actual bong is on the hour (or very close to it).
Ā
The circuit uses an Arduino Nano and I have tried to set the data pins in a logical order as it uses almost all of them to limit confusion.

Schematics
Ā
Chiming Clock Scheme
Circuit diagram

Chimes
unzip to an empty directory and drag the files to an empty micro sdcard one by one .. first one first.. last one last
Code
Ā
Chiming Clock
Arduino
Program using Arduino IDE
#include <Arduino.h>
#include <SoftwareSerial.h>
#include <DFRobotDFPlayerMini.h>
#include <Ds1302.h>
#include <Adafruit_PCD8544.h> // include adafruit PCD8544 (Nokia 5110) library
#include <LowPower.h>
SoftwareSerial mySoftwareSerial(3, 2); // RX, TX
DFRobotDFPlayerMini myDFPlayer;
// DS1302 RTC instance
Ds1302 rtc(6,4,5); //
// Nokia 5110 LCD module connections CLK, DIN, D/C, CS, RST (opposite to the actual pin positions !!)
Adafruit_PCD8544 display = Adafruit_PCD8544(7,8,9,10,11);
const static char* WeekDays[] =
{
" Mon ",
" Tues ",
" Wed ",
" Thurs ",
" Fri ",
" Sat ",
" Sun "
};
const static char* Months[] =
{
"Jan ",
"Feb ",
"Mar ",
"Apr ",
"May ",
"Jun ",
"Jul ",
"Aug ",
"Sep ",
"Oct ",
"Nov ",
"Dec "
};
int hour2;
void setup() {
// Serial.begin(115200);
display.begin(); // initialise the Nokia display
display.clearDisplay(); // clears the screen and buffer
display.setContrast(50);
mySoftwareSerial.begin(9600);
myDFPlayer.begin(mySoftwareSerial);
myDFPlayer.volume(15);
rtc.init(); // initialize the RTC
display.drawRect(0, 0, 84, 30, BLACK);
display.drawRect(0, 29, 84, 12, BLACK);
}
void loop()
{
// get the current time
Ds1302::DateTime now;
rtc.getDateTime(&now);
static uint8_t last_second = 0;
if (last_second != now.second)
{
// Serial.println(hour2);
if ((now.minute == 59) && (now.second == 40)) {
hour2 = now.hour;
hour2++;
// Serial.println("Go to chime");
chime();
}
last_second = now.second;
display.setTextColor(BLACK);
display.setTextSize(2);
display.setCursor(6,10);
if (now.hour <= 9) { //If Hour is single figures, put a 0 in front
display.print("0");
}
display.print(now.hour);
display.print(":");
if (now.minute <= 9) { //If Minute is single figures, put a 0 in front
display.print("0");
}
display.print(now.minute);
// display.print(":");
display.setTextSize(1);
if (now.second <= 9) { //If Seconds is single figures, put a 0 in front
display.print("0");
}
display.print(now.second);
display.setCursor(0,31);
display.print(WeekDays[now.dow -1]);
display.print(now.day);
display.print(" ");
display.print(Months[now.month -1]);
display.display();
display.setTextColor(WHITE);
display.setCursor(0,31);
display.print(WeekDays[now.dow -1]);
display.print(now.day);
display.print(" ");
display.print(Months[now.month -1]);
display.setTextSize(2);
display.setCursor(6,10);
if (now.hour <= 9) {
display.print("0");
}
display.print(now.hour);
display.print(":");
if (now.minute <= 9) {
display.print("0");
}
display.print(now.minute);
// display.print(":");
display.setTextSize(1);
if (now.second <= 9) {
display.print("0");
}
display.print(now.second);
// No need to display the screen again
}
// delay(100);
LowPower.powerDown(SLEEP_250MS,ADC_OFF,BOD_OFF);
}
void chime() {
switch (hour2) {
case 6:
myDFPlayer.play(6);
break;
case 7:
myDFPlayer.play(7);
break;
case 8:
myDFPlayer.play(8);
break;
case 9:
myDFPlayer.play(9);
break;
case 10:
myDFPlayer.play(10);
break;
case 11:
myDFPlayer.play(11);
break;
case 12:
myDFPlayer.play(12);
break;
case 13:
myDFPlayer.play(1);
break;
case 14:
myDFPlayer.play(2);
break;
case 15:
myDFPlayer.play(3);
break;
case 16:
myDFPlayer.play(4);
break;
case 17:
myDFPlayer.play(5);
break;
case 18:
myDFPlayer.play(6);
break;
case 19:
myDFPlayer.play(7);
break;
case 20:
myDFPlayer.play(8);
break;
case 21:
myDFPlayer.play(9);
break;
case 22:
myDFPlayer.play(10);
break;
}
}
The article was first published in hackster, Jun 10, 2022
cr: https://www.hackster.io/stevie135s/chiming-clock-d25797
author: stevie135s
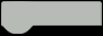