Android app controlled DFPlayer Mini using esp32 Wroom

Things used in this project
Hardware components
Story
Now with Folder Selection ::
I couldn't find a royalty free app to control the DFPlayer Mini from my mobile phone using bluetooth so I went off to create one.
There's very little to wire up really. The Serial 2 lines (16 RX and 17 TX) from the esp32 wroom the power and the speaker.
The 5v in on the esp32 isn't necessarily 5v out so the power to the dfplayer could be connected to 3.3v while it is connected to the PC.
I've included the apk file for android phones. download and install. There's no working version for Apple phones.
The bluetooth connection between the esp32 and the phone should be made (paired) before the app can find and use it. There will be a pop up menu to select the esp broadcast name. that the phone is connected to. I have called the connection "esp32" in this example.
I've updated the functions to include folder selection 1 - 10.
To use folder selection, a blank SD Card needs to be prepared with folders with names 01 to 10.. they can contain mp3's or other folders containing mp3's within them with file names beginning with 0001_
Note : It will not work if you merely rename folders on the SD Card :
Custom parts and enclosures
Folder Version
Download and install on Android Phone
Schematics

Code
Folder Version
Arduino
Flash the esp32 using arduino IDE
#include <BluetoothSerial.h>
#include <Arduino.h>
#include <DFRobotDFPlayerMini.h>
DFRobotDFPlayerMini myDFPlayer;
BluetoothSerial SerialBT;
#define BT_DISCOVER_TIME 10000
int action = 0;
int Song = 0;
static bool btScanAsync = true;
static bool btScanSync = true;
void btAdvertisedDeviceFound(BTAdvertisedDevice* pDevice) {
Serial.printf("Found a device asynchronously: %s\n", pDevice->toString().c_str());
}
void setup() {
Serial2.begin(9600);
Serial.begin(115200);
if (!myDFPlayer.begin(Serial2)) { //Serial2 to communicate with mp3.
Serial.println(F("Unable to begin:"));
Serial.println(F("1.Please recheck the connection!"));
Serial.println(F("2.Please insert the SD card!"));
while(true);
delay(0); // Code to compatible with ESP8266 watch dog.
}
Serial.println(F("DFPlayer Mini online."));
myDFPlayer.setTimeOut(500); //Set serial communictaion time out 500ms
SerialBT.begin("ESP32"); //Bluetooth device name
Serial.println("The device started, now you can pair it with bluetooth!");
if (btScanAsync) {
Serial.print("Starting discoverAsync...");
if (SerialBT.discoverAsync(btAdvertisedDeviceFound)) {
Serial.println("Findings will be reported in \"btAdvertisedDeviceFound\"");
delay(10000);
Serial.print("Stopping discoverAsync... ");
SerialBT.discoverAsyncStop();
Serial.println("stopped");
} else {
Serial.println("Error on discoverAsync f.e. not workin after a \"connect\"");
}
}
if (btScanSync) {
Serial.println("Starting discover...");
BTScanResults *pResults = SerialBT.discover(BT_DISCOVER_TIME);
if (pResults)
pResults->dump(&Serial);
else
Serial.println("Error on BT Scan, no result!");
}
myDFPlayer.volume(10);
}
void loop() {
action = SerialBT.read();
if (action != -1) {
Serial.println (action); //Print what we receive
switch (action) {
case 1:
myDFPlayer.previous(); //Play previous mp3
break;
case 2:
myDFPlayer.pause(); //Stop
Song = 0;
break;
case 3:
if (Song == 0) {
myDFPlayer.play(1); //Play from beginning
myDFPlayer.enableLoopAll();
Song = 1;
}
else {
myDFPlayer.start(); //Play from Pause
myDFPlayer.disableLoopAll();
}
break;
case 4:
myDFPlayer.pause(); //Pause
break;
case 5:
myDFPlayer.next(); //Next
break;
case 6:
myDFPlayer.volumeDown(); //Volume +
break;
case 7:
myDFPlayer.volumeUp(); //Volume -
break;
case 8:
myDFPlayer.EQ(DFPLAYER_EQ_NORMAL);
break;
case 9:
myDFPlayer.EQ(DFPLAYER_EQ_POP);
break;
case 10:
myDFPlayer.EQ(DFPLAYER_EQ_ROCK);
break;
case 11:
myDFPlayer.EQ(DFPLAYER_EQ_JAZZ);
break;
case 12:
myDFPlayer.EQ(DFPLAYER_EQ_CLASSIC);
break;
case 13:
myDFPlayer.EQ(DFPLAYER_EQ_BASS);
break;
case 14:
myDFPlayer.loopFolder(1);
break;
case 15:
myDFPlayer.loopFolder(2);
break;
case 16:
myDFPlayer.loopFolder(3);
break;
case 17:
myDFPlayer.loopFolder(4);
break;
case 18:
myDFPlayer.loopFolder(5);
break;
case 19:
myDFPlayer.loopFolder(6);
break;
case 20:
myDFPlayer.loopFolder(7);
break;
case 21:
myDFPlayer.loopFolder(8);
break;
case 22:
myDFPlayer.loopFolder(9);
break;
case 23:
myDFPlayer.loopFolder(10);
break;
}
}
}
The article was first published in hackster, July 5, 2022
cr: https://www.hackster.io/stevie135s/android-dfplayer-mini-bluetooth-control-04071f
author: stevie135s
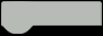