Will guide you to implement a Node-Red-based plant monitoring system on UNIHIKER.
Story
UNIHIKER is a single-board computer that features a 2.8-inch touchscreen, Wi-Fi, and Bluetooth. It is equipped with a light sensor, accelerometer, gyroscope, and microphone. With a built-in co-processor, it can communicate with various analog/digital/I2C/UART/SPI sensors and actuators.

UNIHIKER brings a whole new experience for developers with its pre-installed software, allowing for an incredibly fast and easy start. You can program the single-board computer using a smartphone, tablet, or PC. It also supports popular coding software like VS Code, Mind+, Node-Red, and more.

UNIHIKER also has a built-in IoT service that allows you to store data through the MQTT protocol and access it via a web browser. You can use the integrated Pin Pong control library to directly control UNIHIKER’s built-in sensors and hundreds of connected sensors and actuators using Python.
This tutorial will show how to implement the plant monitoring system using Node-Red with UNIHIKER.
Step-1: Node-Red Installation
First, connect the UNIHIKER to your PC and just wait for 5 sec to boot up. Once you see the UNIHIKER logo, we are good to go.

Next, open Mind+ and connect to UNIHIKER via SSH. Then install the Node-Red using this command.
【bash <(curl -sL https://raw.githubusercontent.com/node-red/linux-installers/master/deb/update-nodejs-and-nodered)】

Then press enter. First, it will ask for the user input. Just press Y.

Again, it will ask for the user's input press Y.

Next, it will ask for your input to install the raspberry pi nodes, but we don't need them. So, just give No, That's all.

It will start installing all the necessary components. Just wait to finish the installation. Once all is done, it will show the IP address of the node red and log.

That's all we have now installed the Node-Red in the UNIHIKER. Type the following command to start the Node-Red.
【node-red-start】

Now you can see the IP, from which we can access the Node-Red. Here is mine.

Then open that in the browser.

Just insert the Inject and payload nodes and try to deploy that.

Let's see the debug logs.

So, now our Node-Red server is working properly. Let's deploy our plant monitoring system.
Step-2: FireBeetle ESP32S3 Sense Setup.
Here I have connected the moisture sensor to the Fire Beetle 2 boards A0 pin and the DHT11 sensor to the D3 pin.

Here is the complete Arduino sketch. Just upload this sketch to the Fire Beetle 2 board and find the IP address.
#include <WiFi.h>
#include <WiFiClient.h>
#include <WebServer.h>
#include "DHT.h"
#define DHTPIN D2 // Digital pin connected to the DHT sensor
#define DHTTYPE DHT11 // DHT 11
const int potPin = A0;
int potValue = 0;
DHT dht(DHTPIN, DHTTYPE);
String temp;
String hum;
String mois;
String HeatIn;
// Your WiFi credentials.
const char* ssid = "ELDRADO";
const char* password = "amazon123";
WebServer server(80);
void handleRoot() {
server.send(200, "text/plain", "hello from esp32!");
}
void handleNotFound() {
String message = "File Not Found\n\n";
message += "URI: ";
message += server.uri();
message += "\nMethod: ";
message += (server.method() == HTTP_GET) ? "GET" : "POST";
message += "\nArguments: ";
message += server.args();
message += "\n";
for (uint8_t i = 0; i < server.args(); i++) {
message += " " + server.argName(i) + ": " + server.arg(i) + "\n";
}
server.send(404, "text/plain", message);
}
void setup()
{
// Debug console
Serial.begin(115200);
WiFi.begin(ssid, password);
Serial.println("");
// Wait for connection
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.print("Connected to ");
Serial.println(ssid);
Serial.print("IP address: ");
Serial.println(WiFi.localIP());
dht.begin();
server.on("/", handleRoot); // http://localIPAddress/
server.on("/dht-temp", []() // http://localIPAddress/dht-temp
{
int t = dht.readTemperature();
temp = t;
server.send(200, "text/plain", temp);
});
server.on("/dht-hum", []() // http://localIPAddress/dht-hum
{
int h = dht.readHumidity();
hum = h;
server.send(200, "text/plain", hum);
});
server.on("/mois", []() // http://localIPAddress/mois
{
int m = analogRead(potPin);
mois = m;
server.send(200, "text/plain", mois);
});
server.onNotFound(handleNotFound);
server.begin();
Serial.println("HTTP server started");
}
void loop()
{
server.handleClient();
}

Next, just type your (http://localIPAddress/dht-temp) in your browser, now you will see the real-time temp data in the browser.

Similarly, you can try on the /dht-hum and /mois. You will find the humidity and moisture data in the browser.
The next thing is to connect it to the Node-Red to monitor and visualize the data.
Step-3: FireBeetle ESP32S3 Sense + Node-Red Integration.
Open the Node-Red dashboard and navigate to the menu and select the manage pallets.

Then click the install tab and search for the dashboard. And install the pallet.

Next, go to the import flow option. and paste this following JSON script.
[
{
"id": "64f52903c284b545",
"type": "tab",
"label": "Flow 1",
"disabled": false,
"info": ""
},
{
"id": "e6a00cc180f7067b",
"type": "http request",
"z": "64f52903c284b545",
"name": "",
"method": "GET",
"ret": "txt",
"paytoqs": "ignore",
"url": "192.168.1.5/dht-temp",
"tls": "",
"persist": false,
"proxy": "",
"insecureHTTPParser": false,
"authType": "",
"senderr": false,
"headers": [],
"x": 630,
"y": 480,
"wires": [
[
"30a7782ba04b1a60",
"93a4d8468a3b9b97"
]
]
},
{
"id": "691c4dc63f202da8",
"type": "inject",
"z": "64f52903c284b545",
"name": "",
"props": [
{
"p": "payload"
},
{
"p": "topic",
"vt": "str"
}
],
"repeat": "3",
"crontab": "",
"once": false,
"onceDelay": 0.1,
"topic": "",
"payload": "",
"payloadType": "date",
"x": 410,
"y": 540,
"wires": [
[
"e6a00cc180f7067b",
"3392fb2a675e207b",
"45cd657c9f6e8342"
]
]
},
{
"id": "3392fb2a675e207b",
"type": "http request",
"z": "64f52903c284b545",
"name": "",
"method": "GET",
"ret": "txt",
"paytoqs": "ignore",
"url": "192.168.1.5/dht-hum",
"tls": "",
"persist": false,
"proxy": "",
"insecureHTTPParser": false,
"authType": "",
"senderr": false,
"headers": [],
"x": 630,
"y": 540,
"wires": [
[
"48feb7d8a567f7a7",
"4c8c08156f2b17a1"
]
]
},
{
"id": "30a7782ba04b1a60",
"type": "ui_gauge",
"z": "64f52903c284b545",
"name": "",
"group": "a7841362.ae40c",
"order": 1,
"width": 0,
"height": 0,
"gtype": "gage",
"title": "Temperature Data",
"label": "deg C",
"format": "{{value}}",
"min": 0,
"max": "100",
"colors": [
"#00b500",
"#e6e600",
"#ca3838"
],
"seg1": "",
"seg2": "",
"x": 820,
"y": 480,
"wires": []
},
{
"id": "48feb7d8a567f7a7",
"type": "ui_gauge",
"z": "64f52903c284b545",
"name": "",
"group": "a7841362.ae40c",
"order": 1,
"width": 0,
"height": 0,
"gtype": "gage",
"title": "Humidity Data",
"label": "%",
"format": "{{value}}",
"min": 0,
"max": "100",
"colors": [
"#00b500",
"#e6e600",
"#ca3838"
],
"seg1": "",
"seg2": "",
"x": 820,
"y": 540,
"wires": []
},
{
"id": "93a4d8468a3b9b97",
"type": "ui_chart",
"z": "64f52903c284b545",
"name": "",
"group": "a7841362.ae40c",
"order": 2,
"width": 0,
"height": 0,
"label": "Temperature",
"chartType": "line",
"legend": "false",
"xformat": "HH:mm:ss",
"interpolate": "linear",
"nodata": "",
"dot": false,
"ymin": "",
"ymax": "",
"removeOlder": 1,
"removeOlderPoints": "",
"removeOlderUnit": "3600",
"cutout": 0,
"useOneColor": false,
"useUTC": false,
"colors": [
"#1f77b4",
"#aec7e8",
"#ff7f0e",
"#2ca02c",
"#98df8a",
"#d62728",
"#ff9896",
"#9467bd",
"#c5b0d5"
],
"outputs": 1,
"useDifferentColor": false,
"x": 1050,
"y": 480,
"wires": [
[]
]
},
{
"id": "4c8c08156f2b17a1",
"type": "ui_chart",
"z": "64f52903c284b545",
"name": "",
"group": "a7841362.ae40c",
"order": 2,
"width": 0,
"height": 0,
"label": "Humidity",
"chartType": "line",
"legend": "false",
"xformat": "HH:mm:ss",
"interpolate": "linear",
"nodata": "",
"dot": false,
"ymin": "",
"ymax": "",
"removeOlder": 1,
"removeOlderPoints": "",
"removeOlderUnit": "3600",
"cutout": 0,
"useOneColor": true,
"useUTC": false,
"colors": [
"#1f77b4",
"#aec7e8",
"#ff7f0e",
"#33e133",
"#98df8a",
"#d62728",
"#ff9896",
"#9467bd",
"#c5b0d5"
],
"outputs": 1,
"useDifferentColor": false,
"x": 1060,
"y": 540,
"wires": [
[]
]
},
{
"id": "45cd657c9f6e8342",
"type": "http request",
"z": "64f52903c284b545",
"name": "",
"method": "GET",
"ret": "txt",
"paytoqs": "ignore",
"url": "192.168.1.5/mois",
"tls": "",
"persist": false,
"proxy": "",
"insecureHTTPParser": false,
"authType": "",
"senderr": false,
"headers": [],
"x": 630,
"y": 600,
"wires": [
[
"14479c17a7ba9806",
"4ba26a97905023d3"
]
]
},
{
"id": "14479c17a7ba9806",
"type": "ui_gauge",
"z": "64f52903c284b545",
"name": "",
"group": "a7841362.ae40c",
"order": 1,
"width": 0,
"height": 0,
"gtype": "gage",
"title": "Moisture Data",
"label": "",
"format": "{{value}}",
"min": 0,
"max": "1034",
"colors": [
"#00b500",
"#e6e600",
"#ca3838"
],
"seg1": "",
"seg2": "",
"x": 820,
"y": 600,
"wires": []
},
{
"id": "4ba26a97905023d3",
"type": "ui_chart",
"z": "64f52903c284b545",
"name": "",
"group": "a7841362.ae40c",
"order": 2,
"width": 0,
"height": 0,
"label": "Moisture Data",
"chartType": "line",
"legend": "false",
"xformat": "HH:mm:ss",
"interpolate": "linear",
"nodata": "",
"dot": false,
"ymin": "",
"ymax": "",
"removeOlder": 1,
"removeOlderPoints": "",
"removeOlderUnit": "3600",
"cutout": 0,
"useOneColor": true,
"useUTC": false,
"colors": [
"#1f77b4",
"#aec7e8",
"#ff7f0e",
"#33e133",
"#98df8a",
"#d62728",
"#ff9896",
"#9467bd",
"#c5b0d5"
],
"outputs": 1,
"useDifferentColor": false,
"x": 1060,
"y": 600,
"wires": [
[]
]
},
{
"id": "a7841362.ae40c",
"type": "ui_group",
"name": "UNIHIKER Node Lab",
"tab": "2ff36ff5.bdc628",
"order": 1,
"disp": true,
"width": "6",
"collapse": false
},
{
"id": "2ff36ff5.bdc628",
"type": "ui_tab",
"name": "Lab1",
"icon": "dashboard",
"disabled": false,
"hidden": false
}
]
This will open up this flow.

Next, open up the HTTP request node and change the IP address of your esp32.

Do the same for all three HTTP request nodes. Next, just deploy. That's all. everything set.
Next, open the browser, enter the Node-Red server address, and finally add the /ui. Like this.

This will open up the dashboard of your Node-Red server. Now you can see all your data in the web dashboard.

Wrap-Up:

Finally, now we can see all our plant environmental data via Node-Red and UNIHIKER server. Thank you and see you guys in another tutorial.
The article was first published in hackster, July 23, 2023
cr: https://www.hackster.io/pradeeplogu0/unihiker-plant-monitoring-system-with-node-red-20f947
author: Pradeep
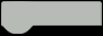