Design a solar radiation meter using Arduino to measure the solar panel’s energy output and irradiance, integrated enhanced performance, and energy management.
Components:
Microcontroller: Arduino Nano (or a similar model) Solar Cell: 0.5V panel (with a short-circuit current of 0.5–1 A) Current Sensor: ACS712 Display: Multispan LCD Display 16x2 with I2C communication protocol Button: For initialization and calibration Resistors: 2 low-value resistors for voltage drop measurement Cabling: Short, thick wires for minimal interference Multispan Energy Management Module (Optional): To track, analyze, and manage energy outputs from solar systems SD Card Module (optional for data logging) Power Supply: 5V regulated power source
Working Principle:
Current Measurement: A solar cell generates current proportional to sunlight intensity. Using the ACS712 current sensor (or Multispan’s current sensor), measure the generated current and convert it into irradiance (W/m²). Voltage Drop: Connect the solar cell to a low-value resistor and measure the voltage drop across the resistor, which gives an indication of the current produced. Data Display: Show the current, power, and voltage data on a Multispan LCD display. Energy Monitoring: Use Multispan energy monitoring solutions to integrate advanced features like device monitoring, historical data tracking, and alarm management. Calibration: On startup, calibrate the system using the button to ensure sensor accuracy.
Code Outline:
/* Solar Radiation Meter with Multispan Integration */
#include
#include
// Pin definitions
const int currentSensorPin = A1; // Pin for current sensor (ACS712 or Multispan sensor)
const int buttonPin = 2; // Pin for calibration button
const int decimalPrecision = 2; // Decimal precision for current display
// Variables
float mVperAmp = 185; // Calibration constant for the ACS712 5A sensor
float current = 0; // Measured current
float irradiation = 0; // Calculated solar radiation (W/m²)
float powerGenerated = 0; // Power output in watts
float accumulatedEnergy = 0; // Accumulated energy in Watt-hours (Wh)
// Multispan I2C LCD (16x2)
LiquidCrystal_I2C lcd(0x27, 16, 2);
void setup() {
pinMode(buttonPin, INPUT_PULLUP); // Button for calibration
lcd.begin();
lcd.backlight();
lcd.print("Solar Meter Init");
delay(2000); // Short delay before starting calibration
lcd.clear();
calibrateSensor();
}
void loop() {
// Read current from sensor
current = readCurrent();
// Calculate solar irradiance (assuming panel area = 1 m²)
irradiation = calculateIrradiation(current);
// Calculate power output in watts
powerGenerated = calculatePower(current);
// Update accumulated energy over time
accumulatedEnergy += powerGenerated / 3600; // Energy in Watt-hours (Wh)
// Display data on LCD
lcd.setCursor(0, 0);
lcd.print("Current: ");
lcd.print(current, decimalPrecision);
lcd.print("A");
lcd.setCursor(0, 1);
lcd.print("Power: ");
lcd.print(powerGenerated, decimalPrecision);
lcd.print("W");
delay(1000); // Refresh every second
}
// Function to read current from sensor
float readCurrent() {
int sensorValue = analogRead(currentSensorPin);
float voltage = (sensorValue / 1024.0) * 5.0; // Convert to voltage
float current = (voltage - 2.5) / (mVperAmp / 1000); // Convert to current
return current;
}
// Function to calculate solar irradiance
float calculateIrradiation(float current) {
// Formula for irradiance calculation (W/m²)
return current * 1000; // Example, needs adjustment based on panel specs
}
// Function to calculate power output
float calculatePower(float current) {
return current * 5; // Assuming panel voltage of 5V, adjust for real values
}
// Function to calibrate the sensor
void calibrateSensor() {
lcd.clear();
lcd.print("Calibrating...");
// Implement calibration logic here
delay(5000); // Simulate calibration delay
lcd.clear();
lcd.print("Calibration Done");
}
Additional Integration with Multispan:
Advanced Monitoring: Integrate Multispan energy monitoring modules to analyze and manage the data from multiple devices. Data Logging: Add an SD card module for recording long-term irradiance and power output for better data analysis. Web Dashboard: Connect to a web interface to view real-time data using Multispan’s IIoT platform. Historical Data Tracking: Leverage Multispan’s historical data tracking to review solar performance over time.
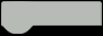