Ultraviolet (UV) Radiation & UV Index
Ultraviolet (UV) radiation is a type of electromagnetic radiation that comes from the sun and artificial sources used in industry, commerce, and recreation. UV radiation can have both positive and negative effects on human health. On the one hand, it stimulates the production of vitamin D in the skin - on the other hand, it can cause skin cancer, premature aging of the skin, cataracts, immune suppression, and other skin disorders. The effects of exposure to UV radiation accumulate over a lifetime. Therefore, it is important to protect yourself from UV radiation by wearing protective clothing, hats, and sunglasses; seeking shade during midday hours; and using sunscreen with an SPF of 30 or higher.
UV radiation can be measured using a device called a UV index meter. A UV index meter measures the intensity of UV radiation in a particular area and provides a numerical value that corresponds to the strength of the UV radiation .
The UV index is a measure of the strength of UV radiation from the sun at a particular place on Earth at a particular time. The UV index is measured on a - color-coded - scale of 0 to 11+, with higher values indicating stronger UV radiation, greater potential for damage to the skin and eye, and less time of exposure neccessary before harm occurs. It is used to help the public plan sun-safe outdoor activities.
The safe UV index depends on the individual's skin type and the duration of exposure. However, as a general rule,
an UV index of 2 or lower is considered safe for all skin types
An UV index of 3 to 5 is considered moderate and requires some protection.
An UV index of 6 to 7 is considered high and requires protection such as a hat, sunglasses, and sunscreen.
An UV index of 8 to 10 is considered very high and requires extra protection such as seeking shade during midday hours and wearing protective clothing.
An UV index of 11 or higher is considered extreme and requires all possible precautions to be taken.
For more information see e.g.,
WHO - Newsroom - Q&A - Radiation: The ultraviolet (UV) index
US EPA - Health Effects of UV Radiation
This project is a quick guide to build a simple UV index meter using the DFRobot ESP32-based FireBeetle 2 Board and the DFRobot Gravity: GUVA-S12SD Analog UV Sensor with the Arduino IoT cloud.
Hardware Setup
FireBeetle 2 ESP32-E IoT Microcontroller: The DFRobot FireBeetle 2 Board is an ESP32-based microcontroller board designed for IoT applications. It is based on the ESP-WROOM-32E module and features dual-core chips that support WiFi and Bluetooth dual-mode communication. The board has a small size, ultra-low power consumption, on-board charging circuit, and easy-to-use interface. See the product page and the documentation for more details.
Gravity: GUVA-S12SD Analog UV Sensor: The Gravity: GUVA-S12SD Analog UV Sensor is a UV sensor that uses a UV photodiode to detect the 240-370nm range of light (which covers UVB and most of UVA spectrum). It can detect the UV wavelength of 200-370nm and has a fast response time with linear analog voltage signal output that changes with the intensity of UV light. The output voltage of the module is proportional to the intensity of the UV light detected by the sensor. The sensor is suitable for detecting the UV radiation in sunlight and can be used in UV Index Monitoring, DIY projects, UV-A Lamp Monitoring, Plants growing Environmental monitoring, etc. See the product page and the documentation for more details.
Connection
The Gravity: GUVA-S12SD Analog UV Sensor comes with one Gravity: Analog Sensor Cable for Arduino with a 3 pin JST connector to connect to the sensor and a servo type connector with pins for signal (blue), GND (black) and PWR (red).
This sensor needs to connected to the FireBeetle 2 Board as follows:
Signal - blue wire - to pin 36 / A0
PWR - red wire - to 3.3V
GND - black wire - to GND
Code
A function read_voltage_mv obtains multiple readings from the analog sensor pin, calculates the average and the resulting voltage in millivolt:
* Function to read sensor output voltage (mV) from
* a GUVA-S12D based analog UV sensor on an ESP32 board.
*/
int read_voltage_mv(int sensor_pin)
{
int sensor_value = 0;
int sensor_value_sum = 0;
int vout = 0;
for (int i = 0; i < 1024; i++)
{
sensor_value = analogRead(sensor_pin);
sensor_value_sum = sensor_value + sensor_value_sum;
delay(1);
}
sensor_value = sensor_value_sum >> 10;
vout = sensor_value * 3260.0 / 4095;
return vout;
}
An other function GUVA_S12SD_uv_index converts the output from the sensor to the corresponding UV index as shown in this table:
/* GUVA_S12SD_uv_index
* Function to convert sensor output voltage (mV) to UV index
* for a GUVA-S12D based analog UV sensor
* See http://www.esp32learning.com/code/esp32-and-guva-s12sd-uv-sensor-example.php
* for conversion table ...
*/
float GUVA_S12SD_uv_index(int vout)
{
const float mv_uvi[12] = {50, 227, 318, 408, 503, 606, 696, 795, 881, 976, 1079, 1170};
int i = 0;
float uvi = 0;
for (i = 0; i < 12; i++)
{
if (vout < mv_uvi[i])
break;
}
if (i < 11)
{
uvi = vout / mv_uvi[i] * (i + 1);
}
else
{
uvi = 11;
}
return uvi;
}
Arduino IoT Cloud & Web Editor
This section describes how to build the UV index meter device with the Arduino IoT Cloud and the Arduino Web Editor
Setup thing & device in Arduino IoT Cloud
1. Sign up and/or log in to www.arduino.cc
2. Open Arduino IoT Cloud, and create a new thing
3. Name the thing and add a variable
4. Define the variable: Name, type, accessibility and update policy
5. Create and associate a new device to the thing:
Click Select Device in the Associated Device section
Click Select Device in the Associated Device section
SET UP NEW DEVICE
Choose Third party device
Check ESP32, search for FireBeetle and choose FireBeetle-ESP32
Name the device
Note the device credentials
6. Setup network connection for the thing: Provide Wi-Fi SID & password and the secret key for the device noted in the previous step
Program and test the device in Arduino Web Editor
1. Open the generated sketch in Arduino Web Editor
Follow the prompts to install and run the Arduino Create Agent, which will provide the following functions:
Identify the Arduino board and other supported devices connected to the computer via USB
Upload the sketch from the Web browser to the board via USB or the network
Read data from the serial monitor, and write data
2. After the board has been connected successfully, modify the generated code by
adding an additional tab and upload the debug2serial.h file from the code section and adding the following lines after the #include "thingProperties.h" line
define DEBUG_SERIAL enabled
#undef DEBUG_SERIAL_VERBOSE
#include "debug2serial.h"
/* Pin for analog sensor */
const int sensorPin = A0;
adding the two functions - read_voltage_mv and GUVA_S12SD_uv_index - introduced earlier - just before the setup function
modifying the loop function as follows:
void loop() {
ArduinoCloud.update();
// Your code here
int vout;
vout = read_voltage_mv(sensorPin);
uv_index = GUVA_S12SD_UV_INDEX(vout);
DEBUG_SERIAL_PRINTF("Sensor GUVA-S12D - Pin %d / Voltage: %d mV\n", sensorPin, vout);
DEBUG_SERIAL_PRINTF("Sensor GUVA-S12D - UV index: %.2f\n", uv_index);
DEBUG_SERIAL_PRINTLN("- - - - - - - - - - - - - - - - - - - - - - - - ");
}
3. Then after compiling and uploading the sketch to the board, open the terminal monitor to check if the setup is working:
4. Go back to the thing's setup page in Arduino IoT Cloud and verify that the device is shown as online the variable has been updated accordingly:
Dashboard & Mobile App
Next step is to setup a dashboard in Arduino IoT Cloud to display the UV index meter readings.
1. Open Arduino IoT Cloud, and create a new dashboard
2. Add a Gauge widget to the dashboard and link it to the uv_index variable of the UV Sensor thing
3. Add a Chart widget to the dashboard and link it to the uv_index variable of the UV Sensor thing
4. Arrange the widgets for screen and mobile view
5. Download and install the Arduino IoT Cloud Remote App, log in into your account and select the UV Sensor thing:
Test
The device was tested at different locations at different times of day. Comparison of the sensor readings with the values published by the local authorities from official weather stations nearby showed that the UV index meter was pretty accurate. The difference was for most readings less than +/- 0.5 depending on the placement of the device.
Field test at the seaside at noon ...
... in a park, late afternoon ...
... and with the device placed on a rooftop in plain sunlight:
Sensor readings shown on the Arduino IoT Remote App ..
... vs. published values for the area on the UV-INDEKS app (Android / iOS)
Resources
Original project on hackster.io: Simple UV Index Meter (FireBeetle 2 & Arduino IoT Cloud)
Complete source code in Arduino Web Editor
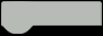