
Popular understanding of the term Diorama denotes a partially three-dimensional model of a landscape showing historical events, nature scenes or cityscapes, for purposes of education or entertainment. What if we mix a micro Vespa with Firebeetle ESP32, a servo motor, wav player, and NTP requests?

Parts required
. Vespa Primavera 1976 model miniature 1/18
. ESP32 Firebeetle
. Micro Servo motor
. 3watts 4 ohms speaker
. HW104 Amp
Circuits
Circuits are easy. Just connect a servo to D2, an audio output to D25. Then use an external 5V power source for the amp. You can leave 5V and GND from Firebeetle to servo. Using a separate power for the amp will give you less noise. HW104w micro amp is used to increase volume before going to the 3 watts speaker.
Even when I like DFPlayer Mini so much, a wav is played right into the Firebeetle without any external modules.

You can use any micro model of a Vespa motorcycle (Harley Davidson or whatever) Just make sure that wheels move. Cut a servo arm and glue it to the wheel. Then you can connect and disconnect the wheel from the servo easily.
// Vespa Clock Diorama
// Roni Bandini @RoniBandini
// Argentina, April 2022
// Amp GPIO 25, GND
// Get Firebeetle from https://www.dfrobot.com/product-2195.html?tracking=60f51c53af980
#include <WiFi.h>
#include <NTPClient.h>
#include <WiFiUdp.h>
#include <Servo.h>
#include "engine.h"
#include "XT_DAC_Audio.h"
Servo servo1;
int servoPin = 2;
int laserPin=14;
int lightOn=0;
int pinAudio=25;
int demoMode=1;
XT_Wav_Class Sound(engine);
XT_DAC_Audio_Class DacAudio(pinAudio,0);
uint32_t DemoCounter=0;
// WiFi
const char* ssid = "SSIHERE";
const char* password = "PASSWORDHERE";
// NTP data
const long utcOffsetInSeconds = -10800; // -3x60x60
char daysOfTheWeek[7][12] = {"Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"};
WiFiUDP ntpUDP;
NTPClient timeClient(ntpUDP, "pool.ntp.org", utcOffsetInSeconds);
void setup() {
servo1.attach(servoPin);
pinMode(laserPin, OUTPUT);
delay(1000);
Serial.begin(115200);
Serial.println("Vespa Diorama started...");
Serial.print(" Connecting to ");
Serial.println(ssid);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
// reset wheel position
servo1.write(180);
timeClient.begin();
delay(2000);
}
void wheel1(){
Serial.println("Wheel forward");
for(int posDegrees = 180; posDegrees >= 0; posDegrees--) {
servo1.write(posDegrees);
delay(10);
}
}
void wheel2(){
Serial.println("Wheel back");
for(int posDegrees = 0; posDegrees <= 180; posDegrees++) {
servo1.write(posDegrees);
delay(50);
}
}
void engineWheel(){
Serial.println("Engine and wheel");
int posDegrees=180;
// forward
while (posDegrees>=0)
{
DacAudio.FillBuffer();
if(Sound.Playing==false)
DacAudio.Play(&Sound);
servo1.write(posDegrees);
posDegrees--;
delay(20);
// wait until wav ends
if (posDegrees==1){
int myCounter=1;
while (myCounter<19){
DacAudio.FillBuffer();
if(Sound.Playing==false)
DacAudio.Play(&Sound);
delay(20);
myCounter++;
}
}
}
}
void loop() {
timeClient.update();
Serial.print(daysOfTheWeek[timeClient.getDay()]);
Serial.print(", ");
Serial.print(timeClient.getHours());
Serial.print(":");
Serial.print(timeClient.getMinutes());
Serial.print(":");
Serial.println(timeClient.getSeconds());
if (timeClient.getMinutes()==0 or demoMode==1){
Serial.print("Hour changed, engine");
engineWheel();
// put wheel back
delay(4000);
wheel2();
delay(4000);
} // 0 minutes
}
Just make sure to edit WiFi credentials since the code needs to be able to connect to your WIFI in order to get NTP hour. You will also have to install #include "XT_DAC_Audio.h" library.
Regarding engine sound. Below you can find the file. Unzip and place into the same folder as the .ino file
Wheel movement
To connect the wheel to the servo just cust one servo arm connector and glue it to the wheel as shown below.


If you want to get the .gcode file for the base, you can find it in my Thingiverse
If you want to change the engine sound, which was obtained from a real Vespa by the way, use the following procedure:
First step is to get a wav file. You can use something like https://x2download.com/en52 to get an audio from a YouTube video.
Then you can use free Audacity to cut the file and convert to WAV. File should be small to fit ESP RAM so change Project Rate 8000Hz. Then Export as Unsigned 8 bit PCM.
Now you have to open the file with Hex editor like https://mh-nexus.de/en/hxd/ Open the wav, Ctrl + A, then Edit Copy as C into a text file named vespa.h
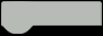