A complete RFID door lock with display!
Things used in this project
Hardware components
Story
https://photos.app.goo.gl/fPhF8hjb4CV2x2xn9
Version 2 is already on the way, with way more features!! Please consider buying the components through the included product links to support this and other projects!
Schematics

Code
Door Lock Code
Arduino
CODE
#include <LiquidCrystal_I2C.h>
#include <SPI.h>
#include <MFRC522.h>
#include <Wire.h>
LiquidCrystal_I2C lcd(0x27, 16, 2);
unsigned long startMillis; //set up millis for reset
unsigned long currentMillis; //pull up millis for reset
const unsigned long period = 604800000; //the value is a number of milliseconds, ie 7 days
#define SS_PIN 10
#define RST_PIN 9
#define RELAY 3 //relay pin
#define BUZZER 2 //buzzer pin
MFRC522 mfrc522(SS_PIN, RST_PIN); // Create MFRC522 instance.
void setup() {
Serial.begin(9600); // Initiate a serial communication
SPI.begin();
mfrc522.PCD_Init();
pinMode(RELAY, OUTPUT);
pinMode(BUZZER, OUTPUT);
noTone(BUZZER);
lcd.init();
lcd.clear();
lcd.backlight();
tone(BUZZER, 1000);
delay(100);
noTone(BUZZER);
lcd.print(F("ARDUINO SECURITY"));
lcd.setCursor(0, 1);
lcd.print(F(" DOOR "));
delay(2000);
lcd.clear();
lcd.print(F(" ACTIVATING "));
lcd.setCursor(0, 1);
lcd.print(F(" SYSTEM "));
delay(2000);
lcd.clear();
digitalWrite(RELAY, HIGH);
delay(2);
digitalWrite(RELAY, LOW);
lcd.print(" PLEASE BE ");
lcd.setCursor(0, 1);
lcd.print(" PATIENT ");
delay(2000);
digitalWrite(RELAY, LOW);
tone(BUZZER, 1000);
delay(1000);
noTone(BUZZER);
lcd.clear();
lcd.print(" SCAN CARD ");
lcd.setCursor(0, 1);
lcd.print(" TO UNLOCK ");
}
void(*resetFunc) (void) = 0;
void loop() {
currentMillis = millis(); //get the current "time" (actually the number of milliseconds since the program started)
if (currentMillis - startMillis >= period) //test whether the period has elapsed
{
resetFunc(); //call reset
}
if ( ! mfrc522.PICC_IsNewCardPresent()) return; // Look for new cards
if ( ! mfrc522.PICC_ReadCardSerial()) return; // Select one of the cards
Serial.print(F("UID tag :"));
String content = "";
byte letter;
for (byte i = 0; i < mfrc522.uid.size; i++)
{
Serial.print(mfrc522.uid.uidByte[i] < 0x10 ? " 0" : " ");
Serial.print(mfrc522.uid.uidByte[i], HEX);
content.concat(String(mfrc522.uid.uidByte[i] < 0x10 ? " 0" : " "));
content.concat(String(mfrc522.uid.uidByte[i], HEX));
}
Serial.println();
content.toUpperCase();
if ((content.substring(1) == "93 6D BE 0D")
|| (content.substring(1) == "51 67 DC B0")
|| (content.substring(1) == "A3 23 74 11") //change here the UID of the card/cards that you want to give access to
|| (content.substring(1) == "55 42 2B 0D"))
{
lcd.clear();
lcd.backlight();
lcd.print(" ACCESS ");
lcd.setCursor(0, 1);
lcd.print(" GRANTED! ");
digitalWrite(RELAY, HIGH);
tone(BUZZER, 1000);
delay(400);
noTone(BUZZER);
delay(6600);
digitalWrite(RELAY, LOW);
lcd.clear();
lcd.print(" SCAN CARD ");
lcd.setCursor(0, 1);
lcd.print(" TO UNLOCK ");
}
else {
lcd.clear();
lcd.backlight();
lcd.print(" ACCESS ");
lcd.setCursor(0, 1);
lcd.print(" DENIED ");
tone(BUZZER, 1000);
delay(150);
noTone(BUZZER);
delay(25);
tone(BUZZER, 1000);
delay(150);
noTone(BUZZER);
delay(25);
tone(BUZZER, 1000);
delay(150);
noTone(BUZZER);
delay(25);
tone(BUZZER, 1000);
delay(150);
noTone(BUZZER);
delay(25);
tone(BUZZER, 1000);
delay(150);
noTone(BUZZER);
delay(1000);
lcd.clear();
lcd.print(" SCAN CARD ");
lcd.setCursor(0, 1);
lcd.print(" TO UNLOCK ");
}
}
The article was first published in hackster, July 30, 2022
cr: https://www.hackster.io/cowboydaniel/arduino-rfid-security-system-2947fd
author: cowboydaniel
License 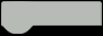
All Rights
Reserved
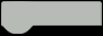
0
More from this category