I am an English Teacher in Thailand. My students must talk for three to five minutes in English. This is one of my first projects. A fun countdown timer to motivate them to enjoy talking in front fo the class. It is a work in progress and one of my first projects.
CODE
#include <Wire.h>
#include <Adafruit_GFX.h>
#include <Adafruit_SSD1306.h>
#include <DFRobot_PAJ7620U2.h>
#define SCREEN_WIDTH 128
#define SCREEN_HEIGHT 64
#define OLED_RESET -1
Adafruit_SSD1306 display(SCREEN_WIDTH, SCREEN_HEIGHT, &Wire, OLED_RESET);
DFRobot_PAJ7620U2 gestureSensor;
int ledPins[] = {27, 26, 14, 12, 25, 13};
int numLeds = sizeof(ledPins) / sizeof(int);
int currentLed = 0;
int currentRound = 0;
unsigned long lastBlinkTime = 0;
bool isRunning = false;
DFRobot_PAJ7620U2 paj;
void setup() {
Serial.begin(115200);
Serial.println("Gesture recognition system base on PAJ7620U2");
while(paj.begin() != 0){
Serial.println("initial PAJ7620U2 failure! Please check if all the connections are fine, or if the wire sequence is correct?");
delay(10);
}
Serial.println("PAJ7620U2init completed, start to test the gesture recognition function");
paj.setGestureHighRate(true);
if(!display.begin(SSD1306_SWITCHCAPVCC, 0x3C)) {
Serial.println(F("SSD1306 allocation failed"));
for(;;);
}
display.clearDisplay();
display.setTextSize(4);
display.setTextColor(WHITE);
display.setCursor(0,0);
display.println("Ready");
display.display();
for(int i=0; i<numLeds; i++) {
pinMode(ledPins[i], OUTPUT);
digitalWrite(ledPins[i], HIGH);
}
lastBlinkTime = millis();
}
void loop() {
DFRobot_PAJ7620U2::eGesture_t gesture = paj.getGesture();
if (gesture != paj.eGestureNone ){
/* Get the string descritpion corresponding to the gesture number.
* The string description could be
* "None","Right","Left", "Up", "Down", "Forward", "Backward", "Clockwise", "Anti-Clockwise", "Wave",
* "WaveSlowlyDisorder", "WaveSlowlyLeftRight", "WaveSlowlyUpDown", "WaveSlowlyForwardBackward"
*/
String description = paj.gestureDescription(gesture);//Convert gesture number into string description
Serial.println("--------------Gesture Recognition System---------------------------");
Serial.print("gesture code = ");Serial.println(gesture);
Serial.print("gesture description = ");Serial.println(description);
Serial.println();
display.clearDisplay();
display.setTextSize(7);
display.setTextColor(WHITE);
display.setCursor(0,0);
display.println("Go");
display.display();
delay(2000);
display.clearDisplay();
display.setTextSize(9);
display.setTextColor(WHITE);
display.setCursor(50,0);
display.println("0");
display.display();
isRunning = true;
}
if(isRunning) {
if(millis() - lastBlinkTime > 2000) {
lastBlinkTime = millis();
digitalWrite(ledPins[currentLed], LOW);
currentLed++;
if(currentLed >= numLeds) {
currentLed = 0;
currentRound++;
for(int i=0; i<numLeds; i++) {
digitalWrite(ledPins[i], HIGH);
}
display.clearDisplay();
display.setCursor(45,0);
display.setTextSize(8);
display.println(currentRound);
display.display();
display.clearDisplay();
}
}
}
}
HARDWARE LIST
1 DFRobot Gesture Sensor
License 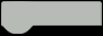
All Rights
Reserved
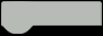
0