DIY Home Security: Arduino Intruder Alarm System with ESP32 & Blynk Cloud Integration
Welcome to this comprehensive tutorial on building your very own Arduino Intruder Alarm System. In this tutorial, we’ll guide you through the process of creating a robust and effective security system using the Beetle ESP32 C3 board, DF robot mm wave Radar (human presence sensor), and Blynk Cloud integration. This project will enable you to monitor and control your security system remotely, receive real-time alerts, and ensure maximum security for your home or workspace.
Components
Â
Beetle ESP32 C3 board: This compact and powerful development board serves as the brain of our Arduino Intruder Alarm System. It provides Wi-Fi connectivity, GPIO pins for interfacing with other components, and easy programming capabilities.Â
DF robot mm wave Radar: The mm wave Radar sensor accurately detects human presence within its detection range. It ensures reliable detection while minimizing false alarms, making it an ideal choice for our project.Â
Buzzer: The buzzer is used as an audible alarm to alert you when an intruder is detected. It provides an additional layer of security by attracting attention and signaling potential threats.Â
LED (Built-in): The built-in LED on the Beetle ESP32 C3 board serves as a visual indicator, providing feedback on the system’s status and the presence of an intruder.Â
Wi-Fi Router: A Wi-Fi router is required to establish a connection between the Arduino board and the Blynk Cloud, enabling remote access and control of the Arduino Intruder Alarm System.
/*
Code for Arduino Intruder Alarm System using ESP32
Created by ArduinoYard.com
*/
/* Comment this out to disable prints and save space */
#define BLYNK_PRINT Serial
/* Fill in information from Blynk Device Info here */
#define BLYNK_TEMPLATE_ID "TMPL6__4LULSh"
#define BLYNK_TEMPLATE_NAME "Intruder Alarm"
#define BLYNK_AUTH_TOKEN "kawepyF-BrlEezuxA8LVduAn6SoYnBg-"
#include <WiFi.h>
#include <WiFiClient.h>
#include <BlynkSimpleEsp32.h>
#include <DFRobot_mmWave_Radar.h>
// Your WiFi credentials.
// Set password to "" for open networks.
char ssid[] = "mySSID";
char pass[] = "myPass";
HardwareSerial mySerial(1); // Using Hardware Serial 1 of the ESP32
DFRobot_mmWave_Radar sensor(&mySerial); // DFrobot mmwave sensor object
#define alarmPin 7
int val = 0;
unsigned long lastMillis = 0;
unsigned long lastEventMillis = 0;
unsigned long updateInterval = 1000; // Interval for updating values on Blynk cloud, min = 1 sec
unsigned long eventInterval = 60000; // Interval for logging event/sending notification, 1 minute
BLYNK_WRITE(V2) {
int alarmVal = param.asInt(); // assigning incoming value from pin V1 to a variable
if (alarmVal == 0) { // If Alarm OFF button pressed on app/web dashboard
digitalWrite(alarmPin, LOW); // Turn OFF alarm
Serial.println("Alarm OFF."); // Print on Serial Monitor
}
else {
digitalWrite(alarmPin, HIGH); // Turn ON alarm
Serial.println("Alarm ON."); // Print on Serial Monitor
}
}
void setup() {
Serial.begin(115200); // Open Serial Monitor at 115200 Baud rate
mySerial.begin(115200, SERIAL_8N1, 20, 21); // RX, TX
pinMode(LED_BUILTIN, OUTPUT); // Set the built-in LED as Output
pinMode(alarmPin, OUTPUT); // Set Alarm pin as output
digitalWrite(alarmPin, LOW); // Turn OFF alarm at start
sensor.factoryReset(); // Restore to the factory settings
sensor.DetRangeCfg(0, 1); // The detection range is as far as 9m
sensor.OutputLatency(0, 0);
Blynk.begin(BLYNK_AUTH_TOKEN, ssid, pass); // Connect to Wi-Fi and Blynk
}
void loop() {
if (millis() - lastMillis >= updateInterval) { // If 1 second has passed, update the values on Blynk cloud
lastMillis = millis(); // Save the update time
Blynk.virtualWrite(V0, val); // Push value on V0
if (val == 1) { // If intruder detected
Blynk.virtualWrite(V1, "Warning! Intruder detected."); // Send a warning status string on V1
digitalWrite(alarmPin, HIGH); // Turn ON alarm if intruder detected
Blynk.virtualWrite(V2, 1); // Push the alarm status on V2
if (millis() - lastEventMillis >= eventInterval) { // If 1 minute has passed since the last intruder notification
lastEventMillis = millis();
Blynk.logEvent("intruderalarm"); // Loga new event and send notification
}
}
else { // If no intruder detected
Blynk.virtualWrite(V1, "No intruder."); // Set the status as normal
}
Blynk.run(); // Update the values on Blynk
}
val = sensor.readPresenceDetection(); // Continuously detect the human presence
digitalWrite(LED_BUILTIN, val); // Turn ON/OFF the built-in LED according to the val
Serial.println(val); // Print the val on Serial monitor
}
// Arduino Intruder Alarm System using ESP32 - END
For Blynk setup follow the video or the following link:
https://arduinoyard.com/arduino-intruder-alarm-system-esp32-blynk/
Conclusion
Congratulations on successfully completing the Arduino Intruder Alarm System project! By following this comprehensive tutorial, you have successfully built a robust security system using the Beetle ESP32 C3 board, DF robot mm wave Radar, and Blynk Cloud integration. Throughout this journey, you have gained valuable knowledge in hardware setup, sensor integration, Blynk Cloud configuration, and Arduino programming.
By implementing the code snippets and customizing the Blynk dashboard, you have created a reliable and user-friendly Arduino Intruder Alarm System. The mm wave Radar sensor accurately detects human presence, allowing the alarm to be activated whenever an intruder is detected. Through the seamless integration with Blynk Cloud, you can remotely monitor and control the security system using the Blynk app, providing you with real-time status updates and the ability to deactivate the alarm at your convenience.
With the Arduino Intruder Alarm System’s completion, you are now equipped with the skills and understanding to explore further possibilities in the fascinating world of Arduino and IoT projects. Share your achievements with the community, engage in discussions, and inspire others to embark on their own security system projects.
We extend our gratitude for joining us on this rewarding journey to create a reliable and intelligent Arduino Intruder Alarm System. Stay tuned for more exciting projects and tutorials as you continue to explore the vast potential of Arduino and IoT technologies.
Remember, the skills and knowledge you have acquired can be applied to various other projects, empowering you to create innovative solutions that enhance security, automation, and connectivity in your surroundings.
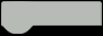